用torch解决问题:使用housing_renamed.csv中的数据构建两个不同的神经网络模型预测value_per_sq_ft:使用3折交叉验证来评价这两个不同的模型的好坏。
时间: 2024-02-13 18:06:53 浏览: 74
好的,我可以帮助你回答这个问题。
首先,我们需要导入必要的库和数据:
```python
import torch
import pandas as pd
from sklearn.model_selection import KFold
from sklearn.preprocessing import StandardScaler
from torch import nn, optim
# 读取数据
df = pd.read_csv('housing_renamed.csv')
# 特征和目标值
features = df.drop(['value_per_sq_ft'], axis=1).values
target = df['value_per_sq_ft'].values
```
然后,我们需要定义两个不同的神经网络模型。这里,我们可以使用两个不同的层数、神经元数量和激活函数:
```python
class Model1(nn.Module):
def __init__(self):
super().__init__()
self.linear1 = nn.Linear(13, 64)
self.linear2 = nn.Linear(64, 1)
self.relu = nn.ReLU()
def forward(self, x):
x = self.linear1(x)
x = self.relu(x)
x = self.linear2(x)
return x
class Model2(nn.Module):
def __init__(self):
super().__init__()
self.linear1 = nn.Linear(13, 128)
self.linear2 = nn.Linear(128, 64)
self.linear3 = nn.Linear(64, 1)
self.relu = nn.ReLU()
def forward(self, x):
x = self.linear1(x)
x = self.relu(x)
x = self.linear2(x)
x = self.relu(x)
x = self.linear3(x)
return x
```
接下来,我们需要定义评价函数。这里,我们可以使用均方根误差(RMSE):
```python
def rmse(y_true, y_pred):
return torch.sqrt(torch.mean((y_true - y_pred)**2))
```
然后,我们需要准备数据并进行标准化:
```python
# 准备数据
kf = KFold(n_splits=3, shuffle=True, random_state=42)
# 标准化
scaler = StandardScaler()
features = scaler.fit_transform(features)
```
现在,我们可以进行交叉验证了。对于每个模型和每个折叠,我们将使用均方根误差作为评价指标:
```python
# 交叉验证
for i, (train_index, test_index) in enumerate(kf.split(features)):
print(f'Fold {i+1}')
train_features, train_target = features[train_index], target[train_index]
test_features, test_target = features[test_index], target[test_index]
# 训练模型1
model1 = Model1()
optimizer1 = optim.Adam(model1.parameters(), lr=0.001)
criterion1 = nn.MSELoss()
for epoch in range(100):
optimizer1.zero_grad()
outputs = model1(torch.Tensor(train_features))
loss = criterion1(outputs.squeeze(), torch.Tensor(train_target))
loss.backward()
optimizer1.step()
y_pred1 = model1(torch.Tensor(test_features))
print(f'Model 1 RMSE: {rmse(torch.Tensor(test_target), y_pred1.squeeze())}')
# 训练模型2
model2 = Model2()
optimizer2 = optim.Adam(model2.parameters(), lr=0.001)
criterion2 = nn.MSELoss()
for epoch in range(100):
optimizer2.zero_grad()
outputs = model2(torch.Tensor(train_features))
loss = criterion2(outputs.squeeze(), torch.Tensor(train_target))
loss.backward()
optimizer2.step()
y_pred2 = model2(torch.Tensor(test_features))
print(f'Model 2 RMSE: {rmse(torch.Tensor(test_target), y_pred2.squeeze())}')
```
最后,我们可以比较两个模型的RMSE,并确定哪个模型更好:
```python
# 比较模型
if rmse(torch.Tensor(target), model1(torch.Tensor(features)).squeeze()) < rmse(torch.Tensor(target), model2(torch.Tensor(features)).squeeze()):
print('Model 1 is better than Model 2')
else:
print('Model 2 is better than Model 1')
```
希望这可以帮助你解决问题!
阅读全文
相关推荐
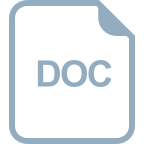
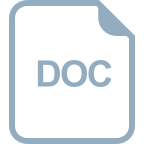
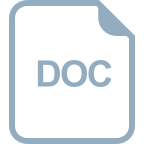

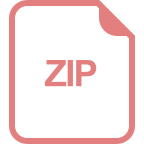
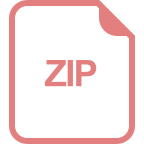
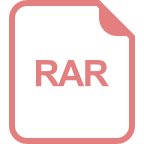
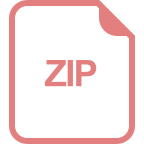
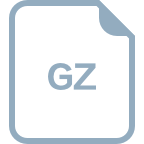
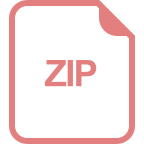
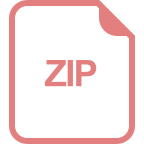
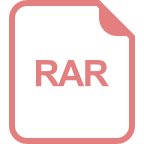
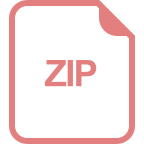
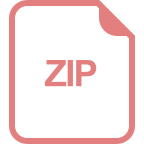
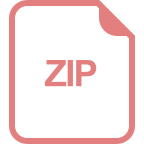
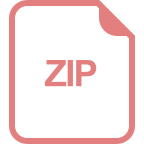
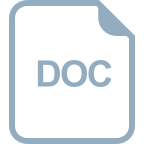
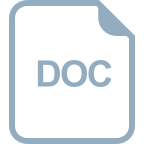