def save(self, name, **kwargs): if not self.save_dir: return if not self.save_to_disk: return data = {} data["model"] = self.model.state_dict() if self.optimizer is not None: data["optimizer"] = self.optimizer.state_dict() if self.scheduler is not None: data["scheduler"] = self.scheduler.state_dict() data.update(kwargs) save_file = os.path.join(self.save_dir, "{}.pth".format(name)) self.logger.info("Saving checkpoint to {}".format(save_file)) torch.save(data, save_file) self.tag_last_checkpoint(save_file)
时间: 2024-02-14 21:11:09 浏览: 17
这是一个保存模型的方法,具体来说:
- `name`:传入一个字符串,表示保存模型的文件名。
- `self.save_dir`:判断模型保存目录是否存在。若不存在,则返回。
- `self.save_to_disk`:判断是否需要将模型保存到磁盘中。若不需要,则返回。
- `data`:创建一个字典,将模型参数、优化器和学习率调度器的状态字典存入其中。
- `save_file`:拼接成最终的保存文件路径。
- `self.logger.info`:记录日志,表示正在保存模型。
- `torch.save`:将 `data` 字典中的内容保存到文件中。
- `self.tag_last_checkpoint`:记录最近一次保存模型的文件路径。
相关问题
def __init__(self, **kwargs):
As an AI language model, I don't have a specific class or object to initialize, but generally speaking, the `__init__` method is a special method in Python classes that is called when an object is created. It is used to initialize the properties or attributes of the object with the values passed as arguments.
The `**kwargs` parameter in the `__init__` method allows you to pass any number of keyword arguments to the constructor. These arguments are collected into a dictionary with the parameter name as the key and the argument value as the value. This provides a flexible way to initialize the object with different sets of properties without having to define a specific constructor for each case.
For example:
```
class Person:
def __init__(self, **kwargs):
self.name = kwargs.get('name', 'John Doe')
self.age = kwargs.get('age', 30)
self.gender = kwargs.get('gender', 'Male')
# Creating an object of Person class with default values
person1 = Person()
# Creating an object of Person class with custom values
person2 = Person(name='Jane Doe', age=25, gender='Female')
```
In the above example, the `Person` class has an `__init__` method that accepts any number of keyword arguments. The method initializes the `name`, `age`, and `gender` properties of the object with the values passed as arguments or with default values if no arguments are provided.
The `person1` object is created with default values for all properties, while the `person2` object is created with custom values for all properties.
def __init__(self, **kwargs): for name in kwargs: setattr(self, name, kwargs[name])
这是一个Python类的构造函数,它使用**kwargs作为输入参数,表示接受任意数量的关键字参数。在函数体内,使用setattr()函数将每个关键字参数赋值给类的属性。这就允许用户在创建类的实例时指定一些属性,并将它们存储到对象中,以便在对象的其他方法中使用。例如,如果你创建了一个类Person,你可以在实例化时传入属性name和age,然后在对象的其他方法中使用这些属性。示例代码如下:
```
class Person:
def __init__(self, **kwargs):
for name in kwargs:
setattr(self, name, kwargs[name])
person1 = Person(name="Alice", age=25)
print(person1.name) # 输出 "Alice"
print(person1.age) # 输出 25
```
相关推荐
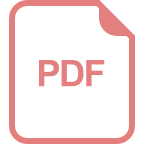
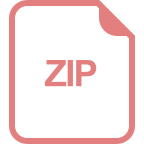
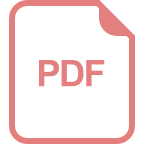













