springboot里面的setURL()应该怎么使用
时间: 2024-04-07 14:33:23 浏览: 4
在Spring Boot中,如果您想设置URL,可以使用Spring的JDBC模块中的数据源配置来实现。具体而言,您可以通过以下步骤来设置URL:
1. 在application.properties或application.yml文件中配置数据源属性。例如,对于PostgreSQL数据库,您可以设置以下属性:
```yaml
spring.datasource.url=jdbc:postgresql://localhost:5432/mydatabase
spring.datasource.username=your-username
spring.datasource.password=your-password
```
2. 在您的代码中,可以通过@Autowired注解将DataSource对象注入到您的类中。例如:
```java
@Autowired
private DataSource dataSource;
```
3. 现在,您可以使用dataSource对象来获取连接并执行数据库操作。例如:
```java
try (Connection connection = dataSource.getConnection()) {
// 执行数据库操作
} catch (SQLException e) {
// 处理异常
}
```
通过这种方式,您可以在Spring Boot应用程序中设置和使用数据库连接URL。请根据您使用的数据库类型和驱动程序进行相应的配置和操作。
相关问题
redis+springboot使用set实现点赞
在redis中,可以使用set数据结构来实现点赞功能。具体实现步骤如下:
1. 定义一个RedisTemplate对象,用于操作redis数据库。
```
@Autowired
private RedisTemplate<String, Object> redisTemplate;
```
2. 定义一个点赞方法,该方法接收两个参数:用户id和文章id。在该方法中,首先判断该用户是否已经点赞过该文章,如果已经点赞,则返回“已经点赞过”;如果未点赞,则将该用户添加到该文章的点赞列表中,并返回“点赞成功”。
```
public String like(String userId, String articleId) {
String key = "article:" + articleId + ":likes";
if (redisTemplate.opsForSet().isMember(key, userId)) {
return "已经点赞过";
} else {
redisTemplate.opsForSet().add(key, userId);
return "点赞成功";
}
}
```
3. 定义一个取消点赞方法,该方法接收两个参数:用户id和文章id。在该方法中,首先判断该用户是否已经点赞过该文章,如果已经点赞,则将该用户从该文章的点赞列表中删除,并返回“取消点赞成功”;如果未点赞,则返回“还未点赞”。
```
public String unlike(String userId, String articleId) {
String key = "article:" + articleId + ":likes";
if (redisTemplate.opsForSet().isMember(key, userId)) {
redisTemplate.opsForSet().remove(key, userId);
return "取消点赞成功";
} else {
return "还未点赞";
}
}
```
4. 在需要使用点赞功能的地方,调用like和unlike方法即可实现点赞功能。
```
String userId = "1001";
String articleId = "2001";
String result = like(userId, articleId);
System.out.println(result);
String result2 = unlike(userId, articleId);
System.out.println(result2);
```
以上就是使用set实现点赞的方法,可以方便地实现点赞功能。
springboot可以set注入bean吗
可以。在Spring Boot中,我们可以使用注解`@Bean`和`@Configuration`来创建一个Bean,并将其注入到其他Bean中。但是,直接使用setter方法注入Bean并不是一个好的实践。我们应该尽可能地使用构造函数注入或者使用`@Autowired`注解来进行依赖注入。这样会使得代码更加可读和可维护。
相关推荐
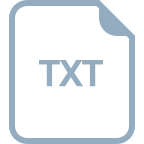












