mybatis plus分页查询
时间: 2023-05-27 11:01:39 浏览: 199
Mybatis Plus提供了非常方便的分页查询功能。具体步骤如下:
1.在mapper中继承BaseMapper,BaseMapper提供了常用的CRUD和分页方法。
```java
public interface UserMapper extends BaseMapper<User> {
}
```
2.在查询方法中使用Page参数,设置分页信息。其中current为当前页码,size为每页记录数
```java
Page<User> page = new Page<>(current, size);
List<User> users = userMapper.selectPage(page, null);
```
3.在xml中可以直接使用 page 参数进行分页查询。同时Mybatis Plus提供了内置的分页插件,可以自动生成分页sql语句,不需要手写。
```xml
<select id="selectUserPage" resultMap="BaseResultMap">
select * from user
</select>
```
相关问题
mybatis plus 分页查询
MyBatis-Plus 是 MyBatis 的增强工具,在 MyBatis 的基础上增加了许多实用的功能,其中包括分页查询。下面是 MyBatis-Plus 分页查询的示例代码:
```java
// 设置分页参数
Page<User> page = new Page<>(1, 10); // 查询第 1 页,每页 10 条记录
// 构造查询条件
QueryWrapper<User> queryWrapper = new QueryWrapper<>();
queryWrapper.eq("status", 1); // 查询状态为 1 的用户
// 执行分页查询
IPage<User> userPage = userService.page(page, queryWrapper); // 执行分页查询
// 输出查询结果
List<User> userList = userPage.getRecords(); // 获取查询结果
long total = userPage.getTotal(); // 获取查询总数
```
上面的代码中,我们首先通过 `new Page<>(1, 10)` 创建一个分页参数对象,表示查询第 1 页,每页 10 条记录。然后,我们使用 `new QueryWrapper<>()` 创建一个查询条件对象,设置查询条件为状态为 1 的用户。最后,我们使用 `userService.page(page, queryWrapper)` 执行分页查询,将查询结果保存到 `userPage` 对象中,然后通过 `userPage.getRecords()` 获取查询结果,通过 `userPage.getTotal()` 获取查询总数。
mybatis plus 分页查询失效
在使用Mybatis Plus进行分页查询时,如果发现分页无法正常发挥作用,这可能是由于配置问题导致的。根据你提供的引用内容,我能够看到你在MybatisPlusConfig类中配置了分页拦截器,但是在双数据源配置类中并没有进行相应的配置。
为了解决这个问题,你需要在数据源配置类中配置分页拦截器。你可以在该类中创建一个MybatisPlusInterceptor对象,并将分页拦截器添加到内部拦截器中。最后,将该拦截器添加到SqlSessionFactory的插件列表中,以使其生效。
通过以上的配置,你就可以保证Mybatis Plus的分页功能正常发挥作用了。请注意,为了避免可能出现的缓存问题,你还需要设置MybatisConfiguration#useDeprecatedExecutor为false。
希望我的回答对你有帮助,如果还有其他问题,请随时提问。
阅读全文
相关推荐
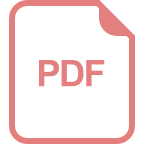
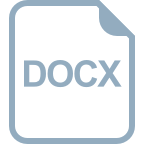
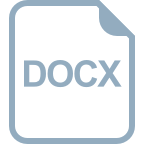












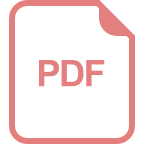