Python实时识别屏幕上与指定图片相似位置
时间: 2024-02-11 07:04:27 浏览: 166
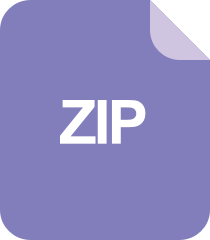
图片相似度识别
这是一个基于特征匹配的Python代码示例,演示如何实时识别屏幕上与指定图片相似的位置。
```python
import cv2
import numpy as np
import pyautogui
# 读取指定图片
template = cv2.imread('template.png', 0)
# 初始化ORB检测器
orb = cv2.ORB_create()
# 提取模板图像的特征点和描述符
kp1, des1 = orb.detectAndCompute(template, None)
while True:
# 获取屏幕截图
screenshot = np.array(pyautogui.screenshot())
# 将截图转换为灰度图像
screenshot_gray = cv2.cvtColor(screenshot, cv2.COLOR_BGR2GRAY)
# 提取截图的特征点和描述符
kp2, des2 = orb.detectAndCompute(screenshot_gray, None)
# 使用FLANN匹配器进行特征点匹配
FLANN_INDEX_LSH = 6
index_params = dict(algorithm=FLANN_INDEX_LSH, table_number=6, key_size=12, multi_probe_level=1)
search_params = dict(checks=50)
flann = cv2.FlannBasedMatcher(index_params, search_params)
matches = flann.knnMatch(des1, des2, k=2)
# 选择最佳匹配
good_matches = []
for m, n in matches:
if m.distance < 0.7 * n.distance:
good_matches.append(m)
if len(good_matches) > 10:
src_pts = np.float32([kp1[m.queryIdx].pt for m in good_matches]).reshape(-1, 1, 2)
dst_pts = np.float32([kp2[m.trainIdx].pt for m in good_matches]).reshape(-1, 1, 2)
# 使用RANSAC算法进行图像变换估计
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
if M is not None:
h, w = template.shape
pts = np.float32([[0, 0], [0, h - 1], [w - 1, h - 1], [w - 1, 0]]).reshape(-1, 1, 2)
dst = cv2.perspectiveTransform(pts, M)
screenshot = cv2.polylines(screenshot, [np.int32(dst)], True, (0, 255, 0), 3, cv2.LINE_AA)
# 显示结果
cv2.imshow('result', screenshot)
# 检测按键
if cv2.waitKey(1) == ord('q'):
break
cv2.destroyAllWindows()
```
这段代码中,首先使用OpenCV的`imread`函数读取指定图片,并使用ORB检测器提取模板图像的特征点和描述符。在每次循环中,提取截图的特征点和描述符,并使用FLANN匹配器进行特征点匹配。然后,选择最佳匹配,并使用RANSAC算法进行图像变换估计,从而找到与指定图片相似的位置。最后,在屏幕上标记出找到的位置,并在窗口中显示结果。
阅读全文
相关推荐


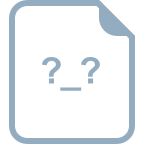
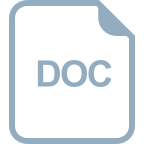
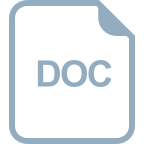
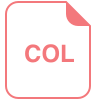
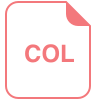
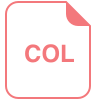
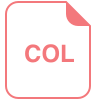
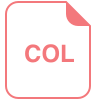
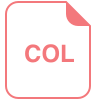
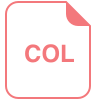
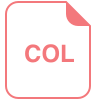
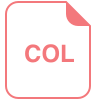
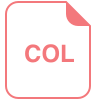