用Java编写:使用同步锁,假设有三个线程(A、B、C)实现线程A执行完了才可以执行线程B,C执行完了再可以执行C,如有四个线程以此类推 启动3个线程 线程1 每一次输入一个字符或字符串,例如A 线程2 每一次输入一个字符或字符串,例如B 线程3 每一次输入一个字符或字符串,例如C 连续3次 要求结果输入是A B C A B C A B C 这种连续的(最后有一个空格)。输入格式: A B C 输出格式: A B C A B C A B C
时间: 2024-02-15 14:03:05 浏览: 106
以下是用Java编写的实现代码:
```java
public class SyncLockExample {
private static int counter = 0;
private static final Object lock = new Object();
public static void main(String[] args) {
Thread threadA = new Thread(() -> {
while (true) {
synchronized (lock) {
while (counter % 3 != 0) {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.print("A ");
counter++;
lock.notifyAll();
}
}
});
Thread threadB = new Thread(() -> {
while (true) {
synchronized (lock) {
while (counter % 3 != 1) {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.print("B ");
counter++;
lock.notifyAll();
}
}
});
Thread threadC = new Thread(() -> {
while (true) {
synchronized (lock) {
while (counter % 3 != 2) {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.print("C ");
counter++;
if (counter % 9 == 0) {
System.out.println();
}
lock.notifyAll();
}
}
});
threadA.start();
threadB.start();
threadC.start();
}
}
```
在这个例子中,使用了一个静态变量 `counter` 来记录当前执行到了第几个线程,使用了一个 `lock` 对象作为同步锁,每个线程在执行时都要先获取 `lock` 的锁。每个线程在执行前,都会先判断 `counter` 的值,如果不满足当前线程应该执行的条件,就会调用 `wait()` 方法释放 `lock` 的锁,并进入等待状态。当 `counter` 的值满足当前线程应该执行的条件时,就会执行当前线程的代码,输出对应的字符,然后将 `counter` 的值加一,并调用 `notifyAll()` 方法通知其他线程可以继续执行了。在输出每个字符后,如果当前字符是 C,并且已经输出了 9 个字符,则输出一个换行符。
阅读全文
相关推荐
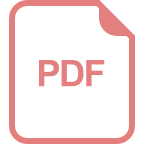
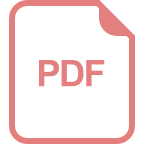
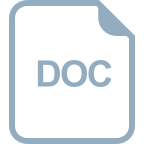
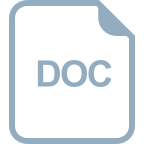
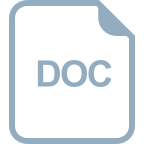
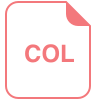
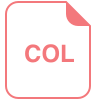
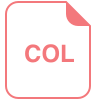
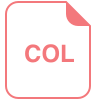
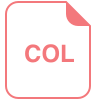
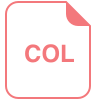
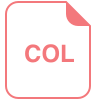
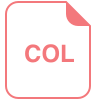
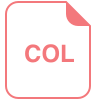
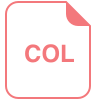
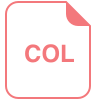
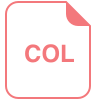
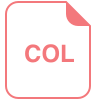