next_permutation请解释一下并举例说明
时间: 2023-09-03 21:08:14 浏览: 76
next_permutation是一个STL算法,它可以将当前排列转换为下一个排列。它的作用是将排列从当前状态改变成字典序下的下一个状态。如果当前排列已经是最后一个排列,next_permutation将返回false,否则返回true。
举个例子:
假设我们有一个排列{1,2,3},它的所有排列如下:
1 2 3
1 3 2
2 1 3
2 3 1
3 1 2
3 2 1
如果我们想从第一个排列(1 2 3)开始,依次输出所有排列,我们可以使用next_permutation算法。具体实现方法如下:
```c++
#include <iostream>
#include <algorithm>
using namespace std;
int main() {
int arr[] = {1, 2, 3};
do {
for (int i = 0; i < 3; i++) {
cout << arr[i] << " ";
}
cout << endl;
} while (next_permutation(arr, arr + 3));
return 0;
}
```
输出结果为:
1 2 3
1 3 2
2 1 3
2 3 1
3 1 2
3 2 1
从上面的输出结果可以看出,next_permutation算法将当前排列转换为了字典序下的下一个状态,直到最后一个排列为止。
相关问题
next_permutation
`next_permutation()` is a C++ STL algorithm that modifies a sequence to its lexicographically next greater permutation. In other words, it rearranges the elements in the sequence to their next greater permutation, if possible, and returns true. If the sequence is already the largest lexicographically, it rearranges it to the smallest lexicographically and returns false.
For example, consider a sequence {1, 2, 3}. The next greater permutation of this sequence is {1, 3, 2}. Similarly, the next greater permutation of {3, 2, 1} is {1, 2, 3}.
The `next_permutation()` algorithm takes two iterators as input: the first iterator points to the beginning of the sequence, and the second iterator points to the end of the sequence. It rearranges the elements in the sequence by swapping adjacent elements until it reaches the next greater permutation, or the sequence is the largest lexicographically.
Here's an example code that demonstrates the usage of `next_permutation()`:
```
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
int main() {
vector<int> v = {1, 2, 3};
do {
for (int i : v) {
cout << i << " ";
}
cout << endl;
} while(next_permutation(v.begin(), v.end()));
return 0;
}
```
In this example, the `do-while` loop prints all the permutations of the vector `v` by repeatedly calling `next_permutation()` until it returns false. The output of this code would be:
```
1 2 3
1 3 2
2 1 3
2 3 1
3 1 2
3 2 1
```
c++next_permutation
### 回答1:
c++中的next_permutation函数是一个STL算法,用于生成下一个排列。它接受两个迭代器作为参数,表示一个范围,然后将该范围中的元素重新排列为下一个字典序更大的排列。如果没有下一个排列,则将范围中的元素重新排列为第一个排列,并返回false。如果存在下一个排列,则返回true。
### 回答2:
c++中的next_permutation函数用于将一组元素重新排列为下一个字典序更大的排列。如果这组元素的当前排列是字典序最大的,那么函数将重新排列为字典序最小的排列。
下面是next_permutation函数的使用方法:
1. 首先,确定要重新排列的元素范围,即指定开始和结束的迭代器。
2. 调用next_permutation函数,传入元素范围的起始和结束迭代器。
3. 函数会将元素重新排列为下一个字典序更大的排列,如果没有下一个更大的排列,函数会重新排列为字典序最小的排列,并返回false。如果有下一个更大的排列,函数会返回true。
4. 使用返回值或迭代器之间的范围确定重新排列后的元素。
下面是一个例子:
```cpp
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> nums = {1, 2, 3};
do {
for (int num : nums) {
std::cout << num << " ";
}
std::cout << std::endl;
} while (std::next_permutation(nums.begin(), nums.end()));
return 0;
}
```
运行结果为:
```
1 2 3
1 3 2
2 1 3
2 3 1
3 1 2
3 2 1
```
上面的代码将打印出给定元素的所有重新排列。在每次迭代时,next_permutation函数将重新排列nums中的元素,并打印出当前的排列。如果有下一个更大的排列,函数将继续循环,直到没有下一个更大的排列为止。
总结:next_permutation函数是在C++中用于生成下一个字典序更大排列的函数,可以通过指定元素范围的迭代器,得到所有可能的重新排列。
### 回答3:
c++中的next_permutation函数用于生成给定序列的下一个全排列。它将当前排列变为下一个较大的排列。如果当前排列是最大排列,则next_permutation将排列调整为最小排列。
next_permutation函数的使用需要包含<algorithm>头文件,并且序列需要已经排序。函数的使用形式为next_permutation(first, last),其中first和last是迭代器,用于表示序列的起始和终止位置。
下面是一个使用next_permutation的示例:
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> nums = {1, 2, 3};
std::sort(nums.begin(), nums.end()); // 首先对序列进行排序
do {
for (int num : nums) {
std::cout << num << " ";
}
std::cout << std::endl;
} while (std::next_permutation(nums.begin(), nums.end())); // 生成下一个全排列,直到生成最后一个全排列
return 0;
}
输出结果为:
1 2 3
1 3 2
2 1 3
2 3 1
3 1 2
3 2 1
在上述示例中,首先对序列进行排序,然后使用do-while循环调用next_permutation生成下一个全排列,并输出每一次生成的排列。当next_permutation返回false时,表示已经生成了最后一个全排列,循环结束。
需要注意的是,序列必须是有序的,否则next_permutation将无法正确生成下一个全排列。另外,next_permutation会改变原有的序列,所以如果需要保留原有序列,请在生成下一个全排列之前保存一份副本。
阅读全文
相关推荐
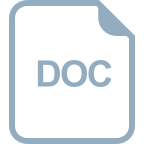
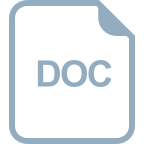
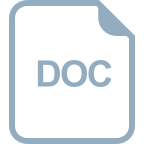
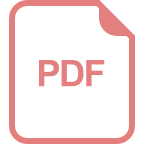
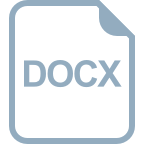





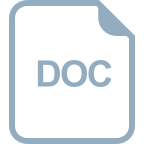
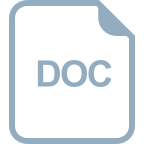
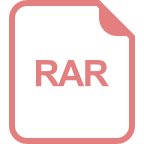
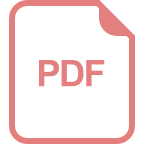
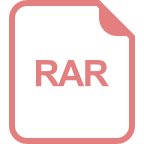
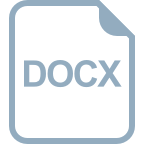