写一个基于gec6818的电子相册显示代码
时间: 2023-09-07 10:15:04 浏览: 48
以下是一个基于GEC6818的电子相册显示代码的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/mman.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <linux/fb.h>
#include <linux/input.h>
#define FB_DEVICE "/dev/fb0"
#define BMP_FILE "example.bmp"
typedef struct BMPFileHeader {
uint16_t fileType; // 文件类型,必须是0x424D,即字符“BM”
uint32_t fileSize; // 整个BMP文件的大小
uint16_t reserved1; // 保留,必须设置为0
uint16_t reserved2; // 保留,必须设置为0
uint32_t bmpOffset; // BMP图像数据的起始位置
} BMPFileHeader;
typedef struct BMPInfoHeader {
uint32_t infoSize; // 本结构体的大小,必须是40
int32_t bmpWidth; // BMP图像的宽度
int32_t bmpHeight; // BMP图像的高度
uint16_t planes; // BMP图像的平面数,必须是1
uint16_t bitCount; // 每个像素的比特数,必须是24
uint32_t compression; // 压缩类型,0表示不压缩
uint32_t imageSize; // BMP图像数据的大小
int32_t xPixelsPerMeter;// 水平分辨率,像素/米
int32_t yPixelsPerMeter;// 垂直分辨率,像素/米
uint32_t colorsUsed; // 实际使用的颜色数,0表示使用所有颜色
uint32_t colorsImportant; // 重要颜色数,0表示所有颜色都重要
} BMPInfoHeader;
int main() {
int fbfd = open(FB_DEVICE, O_RDWR);
if (fbfd == -1) {
perror("open framebuffer device");
exit(EXIT_FAILURE);
}
struct fb_var_screeninfo vinfo;
struct fb_fix_screeninfo finfo;
if (ioctl(fbfd, FBIOGET_VSCREENINFO, &vinfo) == -1) {
perror("get variable screen info");
exit(EXIT_FAILURE);
}
if (ioctl(fbfd, FBIOGET_FSCREENINFO, &finfo) == -1) {
perror("get fixed screen info");
exit(EXIT_FAILURE);
}
size_t screenSize = vinfo.yres * finfo.line_length;
void *fbp = mmap(NULL, screenSize, PROT_READ | PROT_WRITE, MAP_SHARED, fbfd, 0);
if (fbp == MAP_FAILED) {
perror("mmap framebuffer");
exit(EXIT_FAILURE);
}
int bmpfd = open(BMP_FILE, O_RDONLY);
if (bmpfd == -1) {
perror("open BMP file");
exit(EXIT_FAILURE);
}
BMPFileHeader header;
BMPInfoHeader info;
if (read(bmpfd, &header, sizeof(header)) != sizeof(header)) {
perror("read BMP header");
exit(EXIT_FAILURE);
}
if (read(bmpfd, &info, sizeof(info)) != sizeof(info)) {
perror("read BMP info");
exit(EXIT_FAILURE);
}
if (header.fileType != 0x4D42) {
fprintf(stderr, "invalid BMP file type\n");
exit(EXIT_FAILURE);
}
if (info.infoSize != sizeof(info)) {
fprintf(stderr, "invalid BMP info size\n");
exit(EXIT_FAILURE);
}
if (info.bitCount != 24) {
fprintf(stderr, "only support 24-bit BMP files\n");
exit(EXIT_FAILURE);
}
if (lseek(bmpfd, header.bmpOffset, SEEK_SET) == -1) {
perror("seek BMP image data");
exit(EXIT_FAILURE);
}
size_t bmpDataSize = info.imageSize;
void *bmpData = malloc(bmpDataSize);
if (bmpData == NULL) {
perror("allocate BMP data buffer");
exit(EXIT_FAILURE);
}
if (read(bmpfd, bmpData, bmpDataSize) != bmpDataSize) {
perror("read BMP image data");
exit(EXIT_FAILURE);
}
int x, y;
for (y = 0; y < info.bmpHeight; y++) {
for (x = 0; x < info.bmpWidth; x++) {
int pos = (y * info.bmpWidth + x) * 3;
uint8_t b = ((uint8_t *) bmpData)[pos];
uint8_t g = ((uint8_t *) bmpData)[pos + 1];
uint8_t r = ((uint8_t *) bmpData)[pos + 2];
uint32_t color = (r << vinfo.red.offset) | (g << vinfo.green.offset) | (b << vinfo.blue.offset);
*((uint32_t *) (fbp + (y + vinfo.yoffset) * finfo.line_length + (x + vinfo.xoffset) * sizeof(uint32_t))) = color;
}
}
free(bmpData);
munmap(fbp, screenSize);
close(bmpfd);
close(fbfd);
return 0;
}
```
此示例代码打开一个BMP文件,将其解码并显示在GEC6818板子的屏幕上。你可以替换`BMP_FILE`变量的值为你想要显示的BMP文件的路径。注意,此代码仅支持24位BMP文件。
相关推荐
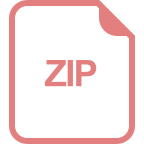














