matlab taylorwin c/c++
时间: 2024-05-02 16:18:22 浏览: 99
Taylorwin is a MATLAB function that computes the coefficients of a Taylor window, which is a type of window function used in signal processing. Here is an example of how to use it in MATLAB:
```matlab
% Define parameters for the Taylor window
M = 64; % window length
beta = 5; % window shape parameter
wtype = 'symmetric'; % window type
% Compute the Taylor window coefficients
w = taylorwin(M, beta, wtype);
% Plot the window
plot(w);
title('Taylor window');
xlabel('Sample index');
ylabel('Amplitude');
```
In C/C++, you can implement the Taylor window function using the following code:
```c
#include <math.h>
void taylorwin(int M, double beta, double *w)
{
int n;
double sum = 0.0, w0;
// Compute the scaling factor for the window
w0 = j1(beta);
// Compute the window coefficients
for (n = 0; n < M; n++) {
double x = (2.0 * n - M + 1.0) / M;
double y = beta * sqrt(1.0 - x * x);
w[n] = j1(y) / (w0 * y);
sum += w[n];
}
// Normalize the window coefficients
for (n = 0; n < M; n++) {
w[n] /= sum;
}
}
```
This code uses the Bessel function `j1` from the `math.h` library to compute the scaling factor and the window coefficients. The `w` array is assumed to be pre-allocated with size `M`. To use this function, you can call it like this:
```c
int M = 64;
double beta = 5.0;
double w[M];
taylorwin(M, beta, w);
// Use the window coefficients
```
阅读全文
相关推荐
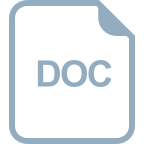
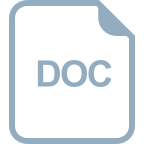
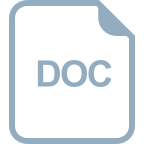











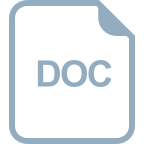
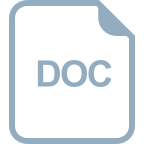
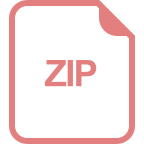
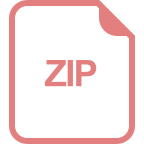
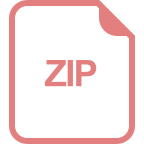