用c++语言编写一个扫雷程序
时间: 2023-09-02 17:07:57 浏览: 122
好的,以下是一个简单的C++语言扫雷程序的代码示例:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
const int BOARD_SIZE = 10;
const int BOMB_COUNT = 5;
void initBoard(int board[][BOARD_SIZE])
{
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
board[i][j] = 0;
}
}
}
void placeBombs(int board[][BOARD_SIZE])
{
int count = 0;
while (count < BOMB_COUNT) {
int x = rand() % BOARD_SIZE;
int y = rand() % BOARD_SIZE;
if (board[x][y] != -1) {
board[x][y] = -1;
count++;
}
}
}
void printBoard(int board[][BOARD_SIZE], bool showBombs = false)
{
cout << " ";
for (int i = 0; i < BOARD_SIZE; i++) {
cout << " " << i;
}
cout << endl;
for (int i = 0; i < BOARD_SIZE; i++) {
cout << i << " ";
for (int j = 0; j < BOARD_SIZE; j++) {
if (showBombs && board[i][j] == -1) {
cout << "* ";
} else if (board[i][j] == -2) {
cout << "F ";
} else if (board[i][j] == -3) {
cout << "? ";
} else if (board[i][j] == -1) {
cout << ". ";
} else {
cout << board[i][j] << " ";
}
}
cout << endl;
}
}
int countAdjacentBombs(int board[][BOARD_SIZE], int x, int y)
{
int count = 0;
if (x > 0 && y > 0 && board[x - 1][y - 1] == -1) {
count++;
}
if (x > 0 && board[x - 1][y] == -1) {
count++;
}
if (x > 0 && y < BOARD_SIZE - 1 && board[x - 1][y + 1] == -1) {
count++;
}
if (y > 0 && board[x][y - 1] == -1) {
count++;
}
if (y < BOARD_SIZE - 1 && board[x][y + 1] == -1) {
count++;
}
if (x < BOARD_SIZE - 1 && y > 0 && board[x + 1][y - 1] == -1) {
count++;
}
if (x < BOARD_SIZE - 1 && board[x + 1][y] == -1) {
count++;
}
if (x < BOARD_SIZE - 1 && y < BOARD_SIZE - 1 && board[x + 1][y + 1] == -1) {
count++;
}
return count;
}
bool isValid(int x, int y)
{
return x >= 0 && x < BOARD_SIZE && y >= 0 && y < BOARD_SIZE;
}
bool isSafe(int board[][BOARD_SIZE], int x, int y)
{
return board[x][y] >= 0 && board[x][y] <= 8;
}
bool hasWon(int board[][BOARD_SIZE])
{
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
if (board[i][j] >= 0 && board[i][j] <= 8) {
return false;
}
}
}
return true;
}
void reveal(int board[][BOARD_SIZE], int x, int y)
{
if (!isValid(x, y) || board[x][y] < 0) {
return;
}
if (board[x][y] > 0 && board[x][y] <= 8) {
board[x][y] = -board[x][y];
return;
}
board[x][y] = -board[x][y];
reveal(board, x - 1, y - 1);
reveal(board, x - 1, y);
reveal(board, x - 1, y + 1);
reveal(board, x, y - 1);
reveal(board, x, y + 1);
reveal(board, x + 1, y - 1);
reveal(board, x + 1, y);
reveal(board, x + 1, y + 1);
}
void flag(int board[][BOARD_SIZE], int x, int y)
{
if (!isValid(x, y) || board[x][y] < 0) {
return;
}
if (board[x][y] == -2) {
board[x][y] = 0;
} else {
board[x][y] = -2;
}
}
void playGame()
{
int board[BOARD_SIZE][BOARD_SIZE];
initBoard(board);
placeBombs(board);
int x, y;
while (true) {
printBoard(board);
cout << "Enter x and y coordinates: ";
cin >> x >> y;
if (!isValid(x, y)) {
cout << "Invalid coordinates. Please try again." << endl;
continue;
}
if (board[x][y] == -1) {
printBoard(board, true);
cout << "You lose!" << endl;
break;
}
if (isSafe(board, x, y)) {
reveal(board, x, y);
if (hasWon(board)) {
printBoard(board, true);
cout << "You win!" << endl;
break;
}
} else {
flag(board, x, y);
}
}
}
int main()
{
srand(time(NULL));
playGame();
return 0;
}
```
该程序实现了一个简单的扫雷游戏,具有以下功能:
- 随机放置指定数量的地雷
- 显示一个由数字和标记组成的游戏板
- 接受用户输入坐标并进行游戏
- 如果用户点击了地雷,游戏结束并显示所有地雷的位置
- 如果用户揭示所有没有地雷的方块,游戏结束并显示所有地雷的位置
- 用户可以标记可能存在地雷的方块,并可以取消标记
请注意,该程序仅作为示例,可能存在漏洞和不足之处,需要根据实际情况进行修改和改进。
阅读全文
相关推荐

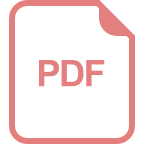
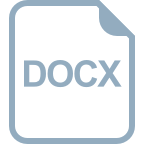
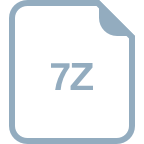
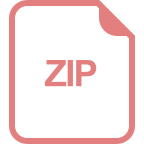


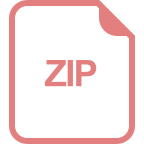
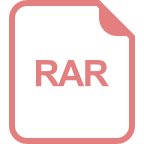
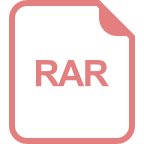
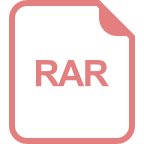
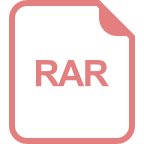
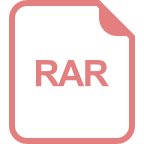
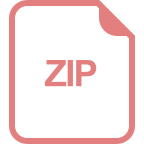
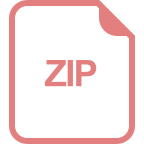
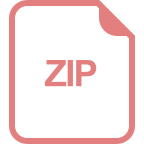