有三个进程P1、P2和C,它们共享一个缓冲区buf。进程P1反复地从设备上读一个记录信息,并将其存入缓冲区buf;进程P2反复地从另一个设备上读一个记录信息,也将其存入缓冲区buf;进程C将缓冲区buf中的记录信息取出,并加工处理。如果缓冲区buf只能存储一个记录,只有在进程C读取信息后,才能存储下一个记录,同时规定,P1或P2不能连续两次向缓冲区buf存放记录,且在初始状态它们中哪一个先向缓冲区buf存放信息都是允许的。请用信号量机制实现进程P1、P2和C的并发执行。
时间: 2024-02-25 14:58:41 浏览: 231
题目描述中提到了缓冲区只能存储一个记录,因此可以使用一个二元信号量来实现对缓冲区的访问控制。同时,在进程P1和P2之间交替存储记录,可以使用两个计数型信号量s1和s2来实现。
具体实现如下:
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h>
#define BUFFER_SIZE 1
int buffer[BUFFER_SIZE];
sem_t mutex, s1, s2;
void *producer1(void *arg) {
int item = 1;
while (1) {
sem_wait(&s1);
sem_wait(&mutex);
buffer[0] = item;
printf("Producer 1 produced item %d\n", item);
item++;
sem_post(&mutex);
sem_post(&s2);
}
}
void *producer2(void *arg) {
int item = 1;
while (1) {
sem_wait(&s2);
sem_wait(&mutex);
buffer[0] = item;
printf("Producer 2 produced item %d\n", item);
item++;
sem_post(&mutex);
sem_post(&s1);
}
}
void *consumer(void *arg) {
int item;
while (1) {
sem_wait(&mutex);
item = buffer[0];
printf("Consumer consumed item %d\n", item);
sem_post(&mutex);
}
}
int main(int argc, char *argv[]) {
sem_init(&mutex, 0, 1);
sem_init(&s1, 0, 1);
sem_init(&s2, 0, 0);
pthread_t tid1, tid2, tid3;
pthread_create(&tid1, NULL, producer1, NULL);
pthread_create(&tid2, NULL, producer2, NULL);
pthread_create(&tid3, NULL, consumer, NULL);
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
pthread_join(tid3, NULL);
sem_destroy(&mutex);
sem_destroy(&s1);
sem_destroy(&s2);
return 0;
}
```
在主函数中创建了三个线程,分别代表P1、P2和C。在每个线程的函数中,使用sem_wait和sem_post函数实现信号量的等待和释放操作。其中,s1和s2分别初始化为1和0,保证了在初始状态下P1或P2中哪一个先向缓冲区buf存放信息都是允许的。mutex初始化为1,保证了对缓冲区的互斥访问。
阅读全文
相关推荐
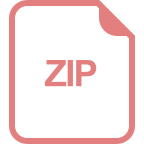
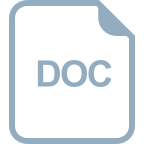
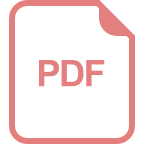
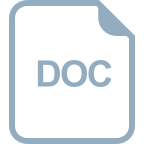
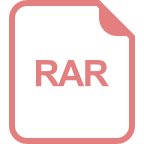
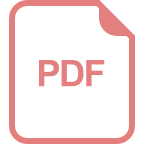
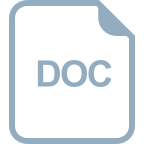
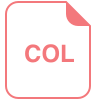
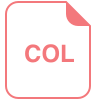
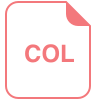







