HttpURLConnection restfull post
时间: 2023-12-18 21:05:42 浏览: 127
下面是使用Java中的HttpURLConnection类构建RESTful API POST请求的简单示例:
```
URL url = new URL("https://jsonplaceholder.typicode.com/posts");
// Create connection object
HttpURLConnection httpURLConnection = (HttpURLConnection) url.openConnection();
// Set request method to POST
httpURLConnection.setRequestMethod("POST");
// Set headers
httpURLConnection.setRequestProperty("Content-Type", "application/json; utf-8");
httpURLConnection.setRequestProperty("Accept", "application/json");
// Enable output and set content length
httpURLConnection.setDoOutput(true);
String postData = "{\"title\": \"My Title\", \"body\": \"My Body\", \"userId\": 1}";
byte[] postDataBytes = postData.getBytes(StandardCharsets.UTF_8);
httpURLConnection.setRequestProperty("Content-Length", String.valueOf(postDataBytes.length));
// Write post data to output stream
try(OutputStream outputStream = httpURLConnection.getOutputStream()) {
outputStream.write(postDataBytes, 0, postDataBytes.length);
}
// Get response code and response message
int responseCode = httpURLConnection.getResponseCode();
String responseMessage = httpURLConnection.getResponseMessage();
// Process response
if (responseCode == HttpURLConnection.HTTP_OK) {
// Read response data
BufferedReader in = new BufferedReader(new InputStreamReader(httpURLConnection.getInputStream()));
String responseData;
StringBuilder responseBuilder = new StringBuilder();
while ((responseData = in.readLine()) != null) {
responseBuilder.append(responseData);
}
in.close();
// Convert response to JSON object
JSONObject jsonObject = new JSONObject(responseBuilder.toString());
System.out.println(jsonObject.toString());
} else {
System.out.println("POST request failed: " + responseMessage);
}
// Disconnect
httpURLConnection.disconnect();
```
在上面的代码中,我们使用HttpURLConnection类创建一个POST请求。我们将JSON对象作为POST数据传递,并将其写入输出流中。然后,我们读取响应并将其转换为JSON对象。
这是一个简单的例子,但你可以根据自己的需求定制它。记得在你的AndroidManifest.xml文件中添加Internet权限。
阅读全文
相关推荐
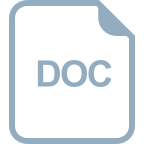
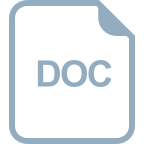
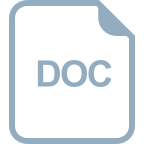
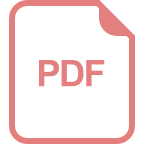







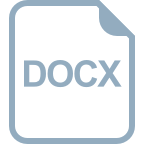
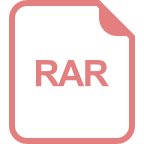





