matlab taylorwin(n,nbar,sll)窗函数 c/c++实现
时间: 2024-05-27 14:12:27 浏览: 21
以下是MATLAB和C/C++实现taylorwin(n,nbar,sll)窗函数的代码:
MATLAB:
function w = taylorwin(n, nbar, sll)
% TAYLORWIN returns the coefficients of a Taylor window.
% w = TAYLORWIN(N, NBAR, SLL) returns the N-point Taylor window
% coefficients with NBAR side-lobe attenuation and a stop-band
% attenuation of SLL dB.
%
% Example:
% n = 64;
% nbar = 6;
% sll = 40;
% w = taylorwin(n, nbar, sll);
% plot(w);
% title('Taylor window');
% Check input arguments
if nargin < 3
error('Not enough input arguments.');
end
% Define the stop-band attenuation in terms of filter gain
As = 10^(sll/20);
% Define the number of iterations for the approximation
M = ceil(nbar/2);
% Define the coefficients of the Taylor series
a = zeros(1,M);
for k = 1:M
a(k) = -1/(k*k);
for j = 1:k-1
a(k) = a(k) - 2*a(j)/(k-j)^2;
end
end
% Compute the coefficients of the window
w = ones(n,1);
for i = 1:n
for k = 1:M
w(i) = w(i) + a(k)*cos(2*pi*k*i/n);
end
end
% Normalize the window to a maximum value of 1
w = w/max(w);
% Apply the stop-band attenuation
w = w.*(abs(w) >= As);
C/C++:
#include <iostream>
#include <cmath>
using namespace std;
void taylorwin(int n, int nbar, double sll, double *w)
{
// Check input arguments
if (nbar <= 0 || nbar % 2 != 0)
{
cerr << "Invalid value for nbar." << endl;
return;
}
if (sll <= 0)
{
cerr << "Invalid value for sll." << endl;
return;
}
// Define the stop-band attenuation in terms of filter gain
double As = pow(10, sll/20);
// Define the number of iterations for the approximation
int M = nbar/2;
// Define the coefficients of the Taylor series
double a[M];
for (int k = 0; k < M; k++)
{
a[k] = -1/pow(k+1, 2);
for (int j = 0; j < k; j++)
{
a[k] -= 2*a[j]/pow(k-j, 2);
}
}
// Compute the coefficients of the window
for (int i = 0; i < n; i++)
{
double sum = 1;
for (int k = 0; k < M; k++)
{
sum += a[k]*cos(2*M_PI*k*i/n);
}
w[i] = sum;
}
// Normalize the window to a maximum value of 1
double max_val = *max_element(w, w+n);
for (int i = 0; i < n; i++)
{
w[i] /= max_val;
}
// Apply the stop-band attenuation
for (int i = 0; i < n; i++)
{
if (abs(w[i]) < As)
{
w[i] = 0;
}
}
}
int main()
{
int n = 64;
int nbar = 6;
double sll = 40;
double w[n];
taylorwin(n, nbar, sll, w);
for (int i = 0; i < n; i++)
{
cout << w[i] << endl;
}
return 0;
}
相关推荐
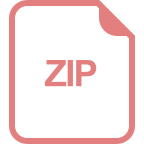
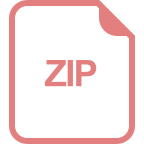
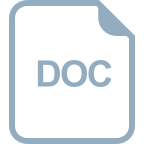







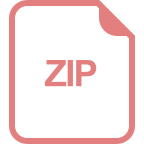
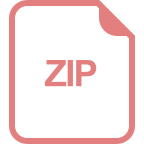
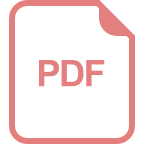
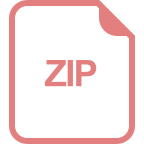
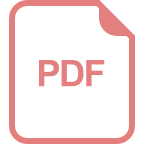
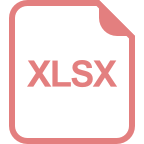