利用python与pyqt5实现多层界面设计
时间: 2023-10-21 21:02:20 浏览: 44
在Python中,PyQt5是一个强大的GUI工具包,可以用于创建各种GUI应用程序,包括多层界面设计。下面是一个简单的示例代码,演示如何使用PyQt5实现多层界面设计:
```python
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QVBoxLayout, QHBoxLayout, QPushButton, QLabel
class MainWindow(QWidget):
def __init__(self):
super().__init__()
# 创建垂直布局
layout = QVBoxLayout()
# 添加标签
label = QLabel("Welcome to the Main Window!", self)
layout.addWidget(label)
# 创建按钮并添加到水平布局
button1 = QPushButton("Open Window 1", self)
button1.clicked.connect(self.open_window1)
hlayout = QHBoxLayout()
hlayout.addWidget(button1)
# 添加水平布局到垂直布局
layout.addLayout(hlayout)
# 设置主窗口布局
self.setLayout(layout)
self.setWindowTitle("Main Window")
def open_window1(self):
self.window1 = Window1()
self.window1.show()
class Window1(QWidget):
def __init__(self):
super().__init__()
# 创建垂直布局
layout = QVBoxLayout()
# 添加标签
label = QLabel("Welcome to Window 1!", self)
layout.addWidget(label)
# 创建按钮并添加到水平布局
button1 = QPushButton("Open Window 2", self)
button1.clicked.connect(self.open_window2)
hlayout = QHBoxLayout()
hlayout.addWidget(button1)
# 添加水平布局到垂直布局
layout.addLayout(hlayout)
# 设置窗口布局
self.setLayout(layout)
self.setWindowTitle("Window 1")
def open_window2(self):
self.window2 = Window2()
self.window2.show()
class Window2(QWidget):
def __init__(self):
super().__init__()
# 创建垂直布局
layout = QVBoxLayout()
# 添加标签
label = QLabel("Welcome to Window 2!", self)
layout.addWidget(label)
# 创建按钮并添加到水平布局
button1 = QPushButton("Close Window 2", self)
button1.clicked.connect(self.close)
hlayout = QHBoxLayout()
hlayout.addWidget(button1)
# 添加水平布局到垂直布局
layout.addLayout(hlayout)
# 设置窗口布局
self.setLayout(layout)
self.setWindowTitle("Window 2")
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
在这个示例代码中,我们创建了三个窗口:主窗口、窗口1和窗口2。主窗口包含一个标签和一个按钮,点击按钮可以打开窗口1。窗口1也包含一个标签和一个按钮,点击按钮可以打开窗口2。窗口2只包含一个标签和一个按钮,点击按钮可以关闭窗口2。
通过这个简单的示例代码,你可以了解如何使用PyQt5实现多层界面设计。你可以根据需求添加更多的窗口和控件,并设计更复杂的GUI应用程序。
相关推荐
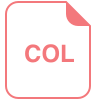
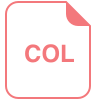
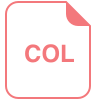
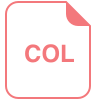
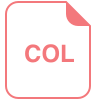







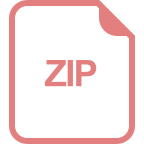
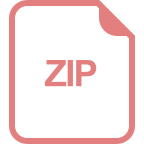
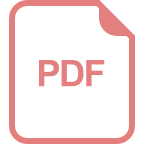