统计字符串中数字字符的个数
时间: 2023-08-26 09:05:36 浏览: 98
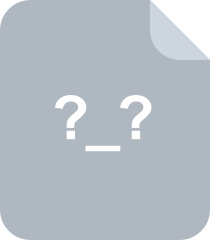
统计一个字符串中数字的个数
好的,下面是使用 C/C++ 实现统计字符串中数字字符的个数的代码:
使用指针:
```c
#include <stdio.h>
int count_digits(char* str){
int count = 0;
while(*str != '\0'){
if(*str >= '0' && *str <= '9'){
count++;
}
str++;
}
return count;
}
int main(){
char str[100];
printf("请输入字符串:\n");
scanf("%s", str);
printf("字符串中数字字符的个数为:%d\n", count_digits(str));
return 0;
}
```
在 `count_digits` 函数中,使用指针遍历字符串中的每一个字符,如果该字符是数字字符,则计数器加一。最后返回计数器的值。
使用引用:
```c++
#include <iostream>
#include <string>
using namespace std;
int count_digits(string& str){
int count = 0;
for(char c : str){
if(c >= '0' && c <= '9'){
count++;
}
}
return count;
}
int main(){
string str;
cout << "请输入字符串:" << endl;
cin >> str;
cout << "字符串中数字字符的个数为:" << count_digits(str) << endl;
return 0;
}
```
在 `count_digits` 函数中,使用 `for` 循环遍历字符串中的每一个字符,如果该字符是数字字符,则计数器加一。最后返回计数器的值。
阅读全文
相关推荐
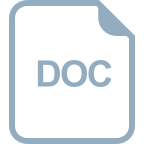











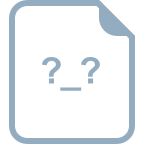
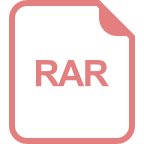