C语言求一个二维数组中零的个数
时间: 2024-01-25 07:03:10 浏览: 34
可以使用双重循环遍历二维数组,统计其中值为0的元素个数即可。具体代码如下:
```c
int countZero(int arr[][N], int rows, int cols) {
int count = 0;
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
if (arr[i][j] == 0) {
count++;
}
}
}
return count;
}
```
其中,`arr`表示二维数组,`rows`表示行数,`cols`表示列数,`count`表示零的个数。函数返回值为零的个数。
相关问题
c语言中求一个二维数组中的0的个数
可以使用循环遍历二维数组的每个元素,统计其中值为0的元素数量,如下所示:
```c
int countZeros(int arr[][N], int rows, int cols) {
int count = 0;
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
if (arr[i][j] == 0) {
count++;
}
}
}
return count;
}
```
其中,`arr`为二维数组,`rows`和`cols`分别表示数组的行数和列数。函数返回数组中0的数量。
c语言求二维数组每列平均数
在C语言中,求二维数组每列的平均数可以通过以下步骤实现:
首先,我们需要定义一个二维数组,并初始化其中的元素。
接下来,我们可以利用两层嵌套的for循环来遍历二维数组,其中外层循环用于遍历列,内层循环用于计算每列的和。
在内层循环中,我们可以累加每列的元素值,并且在累加的过程中记录每列中元素的个数。
计算完每列的和后,我们可以根据每列的元素个数计算该列的平均数。
最后,将每列的平均数打印输出,即可得到二维数组每列的平均数。
下面是一个简单的C语言代码示例:
```c
#include <stdio.h>
int main() {
int array[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
int rows = 3;
int cols = 3;
for (int j = 0; j < cols; j++) {
int sum = 0;
for (int i = 0; i < rows; i++) {
sum += array[i][j];
}
float average = (float)sum / rows;
printf("第%d列的平均数为:%f\n", j+1, average);
}
return 0;
}
```
在上述代码中,我们定义了一个3x3的二维数组array,并对其每列进行了平均数的计算。通过这样的方法,我们可以得到二维数组每列的平均数。
相关推荐
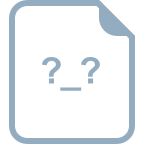
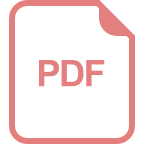
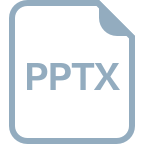












