In a microcontroller based on the 8051, the data memory contains 8 samples from one of 16 possible 12-bit ADC (each ADC is called a channel and has a unique 4-bit channel identifier), in 16 consecutive locations starting at address 30h. Each sample is stored in two consecutive memory locations with the lower 8 bits stored at the lower address and the 4 most significant bits are stored in the upper address along with the associated 4-bit channel number as shown in Figure 1. Together these two locations detail the ADC channel from which the same came from and the 12-bit unsigned number representing the analog voltage presented to that ADC. Write efficient assembly language instructions to calculate the average of the 8 sample values (ignoring the channel numbers), and store the result at address 40h, least significant byte first.
时间: 2024-01-31 08:01:52 浏览: 25
Here is a possible solution in assembly language for calculating the average of the 8 sample values and storing the result at address 40h:
```
MOV R0, #0 ; initialize sum to zero
MOV R1, #30h ; start of data memory
MOV R2, #8 ; number of samples
LOOP:
MOV A, @R1 ; load lower 8 bits of sample
ADD R0, A ; add to sum
INC R1 ; next memory location
MOV A, @R1 ; load upper 4 bits of sample and channel number
SWAP A ; swap nibbles
ANL A, #0Fh ; mask out channel number
ADDC A, #0 ; add carry from swap
INC R1 ; next memory location
DJNZ R2, LOOP ; repeat for all samples
DIV:
MOV A, R0 ; divide sum by 8 (shift right 3 bits)
MOV R3, #8 ; divisor
DIV_LOOP:
RRC A ; shift right with carry
DJNZ R3, DIV_LOOP ; repeat 8 times
MOV @40h, A ; store result at address 40h (least significant byte first)
```
The solution uses three registers: R0 for the sum, R1 for the data memory pointer, and R2 for the loop counter. The loop iterates 8 times, loading each sample value and adding it to the sum. The upper 4 bits of each sample are also loaded and processed to extract the channel number, but it is ignored for the average calculation. After the loop, the sum is divided by 8 using a shift right operation 8 times. Finally, the result is stored at address 40h (least significant byte first).
相关推荐
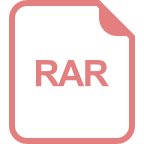
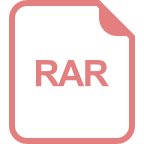














