// 下载器类 class Downloader { constructor(data, options) { this.data = data; this.options = options; this.item = null; this.onStart = null; this.onProgress = null; this.onCompleted = null; this.data.progress = 0; // 新增 progress 属性 this.data.status='pause';//true 表示 进度没有结束,继续下载 this.data.timeid=0; } start() { const win = BrowserWindow.getFocusedWindow(); this.options.onStarted = (item) => { this.item = item; this.data.status='process';//true 表示 进度没有结束,继续下载 this.onStart(); }; this.options.onTotalProgress=(progress)=>{ this.data.progress = progress.percent * 100; // 更新 progress 属性 this.onTotalProgress(progress); } this.options.onCompleted = () => { this.onCompleted(); }; download(win, this.data.dowloadLink, this.options); } pause() { if (this.item) { this.item.pause(); } } resume() { if (this.item) { this.item.resume(); } } cancel() { if (this.item) { this.item.cancel(); } } } 这是我说的类,里面有异步方法,怎么实现
时间: 2024-02-25 15:52:55 浏览: 27
你可以在异步方法执行完毕后,通过 Promise 或回调函数的方式通知外部代码。例如,在 onCompleted 回调函数中设置一个 Promise,然后在外部代码中等待这个 Promise 执行完毕,再执行下一步操作。类似下面这样:
```
class Downloader {
constructor(data, options) {
this.data = data;
this.options = options;
this.item = null;
this.onStart = null;
this.onProgress = null;
this.onCompleted = null;
this.data.progress = 0;
this.data.status = 'pause';
this.data.timeid = 0;
}
start() {
const win = BrowserWindow.getFocusedWindow();
this.options.onStarted = (item) => {
this.item = item;
this.data.status = 'process';
this.onStart();
};
this.options.onTotalProgress = (progress) => {
this.data.progress = progress.percent * 100;
this.onTotalProgress(progress);
};
this.options.onCompleted = () => {
this.onCompletedPromise.resolve(); // 执行 Promise
};
this.onCompletedPromise = {}; // 保存 Promise 对象
this.onCompletedPromise.promise = new Promise((resolve, reject) => {
this.onCompletedPromise.resolve = resolve;
this.onCompletedPromise.reject = reject;
});
download(win, this.data.dowloadLink, this.options);
}
async pause() {
if (this.item) {
await this.onCompletedPromise; // 等待 Promise 执行完毕
this.item.pause();
}
}
async resume() {
if (this.item) {
await this.onCompletedPromise; // 等待 Promise 执行完毕
this.item.resume();
}
}
async cancel() {
if (this.item) {
await this.onCompletedPromise; // 等待 Promise 执行完毕
this.item.cancel();
}
}
}
```
这样就能保证异步方法执行完毕后再执行外部代码了。
相关推荐
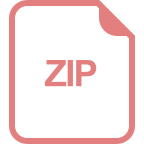
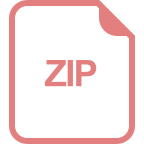





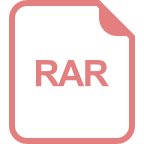
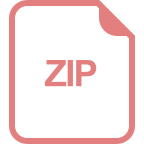
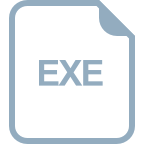
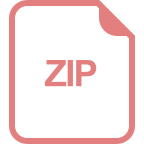
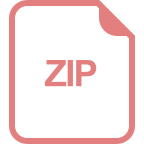
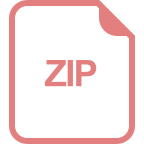