在py文件中用kivy写手机应用,在手机界面按下“开始录音”的按钮时调用百度api开始录音并识别,同时在文本框中展现识别结果,按下”清空”按钮清除文本框内容;同时在界面创建“加载样品溶液”“加载清洗液一““加载清洗液二““加载洗脱溶液”“总操作”的按钮代码生成
时间: 2023-06-20 13:04:20 浏览: 93
以下是一个简单的示例代码,可以实现你所描述的功能:
```python
from kivy.app import App
from kivy.uix.button import Button
from kivy.uix.textinput import TextInput
from kivy.uix.boxlayout import BoxLayout
import os
import json
from aip import AipSpeech
class VoiceRecognitionApp(App):
def __init__(self, *args, **kwargs):
super(VoiceRecognitionApp, self).__init__(*args, **kwargs)
self.text_input = None
def build(self):
layout = BoxLayout(orientation='vertical')
# 创建文本框
self.text_input = TextInput(multiline=True)
layout.add_widget(self.text_input)
# 创建“开始录音”按钮
record_button = Button(text='开始录音')
record_button.bind(on_press=self.start_recording)
layout.add_widget(record_button)
# 创建“清空”按钮
clear_button = Button(text='清空')
clear_button.bind(on_press=self.clear_text_input)
layout.add_widget(clear_button)
# 创建“加载样品溶液”按钮
load_sample_button = Button(text='加载样品溶液')
load_sample_button.bind(on_press=self.load_sample_solution)
layout.add_widget(load_sample_button)
# 创建“加载清洗液一”按钮
load_cleaner1_button = Button(text='加载清洗液一')
load_cleaner1_button.bind(on_press=self.load_cleaner1_solution)
layout.add_widget(load_cleaner1_button)
# 创建“加载清洗液二”按钮
load_cleaner2_button = Button(text='加载清洗液二')
load_cleaner2_button.bind(on_press=self.load_cleaner2_solution)
layout.add_widget(load_cleaner2_button)
# 创建“加载洗脱溶液”按钮
load_elution_button = Button(text='加载洗脱溶液')
load_elution_button.bind(on_press=self.load_elution_solution)
layout.add_widget(load_elution_button)
# 创建“总操作”按钮
total_operation_button = Button(text='总操作')
total_operation_button.bind(on_press=self.total_operation)
layout.add_widget(total_operation_button)
return layout
def start_recording(self, instance):
# 调用百度语音识别API进行语音识别
app_id = 'your_app_id'
api_key = 'your_api_key'
secret_key = 'your_secret_key'
client = AipSpeech(app_id, api_key, secret_key)
result = client.asr(self.record_audio(), 'pcm', 16000, {
'dev_pid': 1536,
})
if result['err_no'] == 0:
# 在文本框中展示识别结果
self.text_input.text += result['result'][0]
else:
print('语音识别失败')
def record_audio(self):
# 调用百度语音识别API进行语音识别
os.system('arecord -D "plughw:1,0" -f S16_LE -r 16000 -d 5 -c 1 my_audio.pcm')
with open('my_audio.pcm', 'rb') as f:
return f.read()
def clear_text_input(self, instance):
# 清空文本框内容
self.text_input.text = ''
def load_sample_solution(self, instance):
# 加载样品溶液
pass
def load_cleaner1_solution(self, instance):
# 加载清洗液一
pass
def load_cleaner2_solution(self, instance):
# 加载清洗液二
pass
def load_elution_solution(self, instance):
# 加载洗脱溶液
pass
def total_operation(self, instance):
# 总操作
pass
if __name__ == '__main__':
VoiceRecognitionApp().run()
```
需要注意的是,以上代码中的语音识别部分使用了百度语音识别API,需要在百度开发者平台上创建应用并获取相应的 App ID、API Key 和 Secret Key。同时,还需要安装 Baidu AIP SDK,可以通过以下命令进行安装:
```
pip install baidu-aip
```
阅读全文
相关推荐
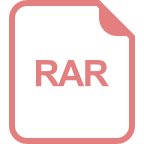
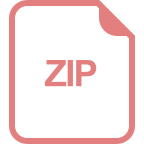
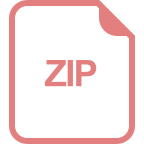
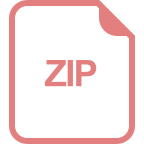
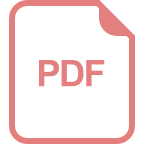
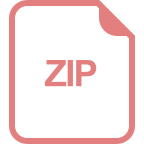
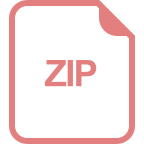
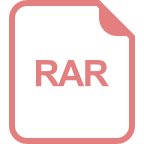
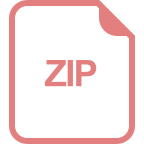
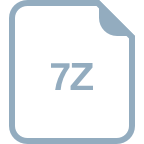
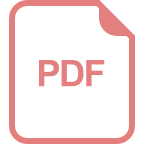
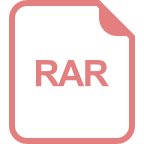
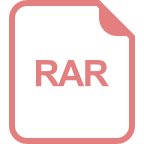
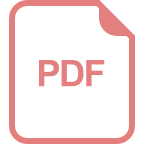
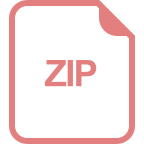
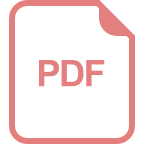