SpringBoot整合Mybatis和Redis原理
时间: 2024-01-22 14:03:48 浏览: 101
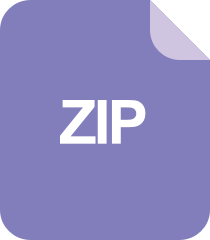
SpringBoot整合Mybatis

SpringBoot整合Mybatis和Redis的原理主要包括以下几个方面:
1. SpringBoot整合Mybatis:SpringBoot提供了Mybatis的自动配置功能,只需要在pom.xml中引入相关的依赖,配置好数据源,就可以使用Mybatis进行数据库操作。
2. SpringBoot整合Redis:SpringBoot提供了对Redis的支持,只需要在pom.xml中引入相关的依赖,配置好Redis的连接信息,就可以使用Redis进行缓存操作。
3. 整合过程:在整合过程中,需要将Mybatis的查询结果进行序列化,存入Redis缓存中,同时在查询数据时,先从Redis缓存中获取数据,如果获取不到再从数据库中获取数据,并将结果存入Redis缓存中。
具体实现可以参考以下步骤:
1. 配置数据源和Mybatis:在application.properties文件中配置数据源和Mybatis相关信息,例如:
```
spring.datasource.url=jdbc:mysql://localhost:3306/test?useSSL=false
spring.datasource.username=root
spring.datasource.password=123456
mybatis.mapper-locations=classpath:mapper/*.xml
```
2. 配置Redis:在application.properties文件中配置Redis相关信息,例如:
```
spring.redis.host=localhost
spring.redis.port=6379
spring.redis.password=
spring.redis.database=0
```
3. 配置RedisTemplate:使用RedisTemplate进行Redis操作,可以在配置类中进行配置,例如:
```
@Configuration
public class RedisConfig {
@Autowired
private RedisConnectionFactory redisConnectionFactory;
@Bean
public RedisTemplate<String, Object> redisTemplate() {
RedisTemplate<String, Object> redisTemplate = new RedisTemplate<>();
redisTemplate.setConnectionFactory(redisConnectionFactory);
redisTemplate.setKeySerializer(new StringRedisSerializer());
redisTemplate.setValueSerializer(new GenericJackson2JsonRedisSerializer());
return redisTemplate;
}
}
```
4. 编写缓存操作代码:在查询数据时,先从Redis缓存中获取数据,如果获取不到再从数据库中获取数据,并将结果存入Redis缓存中,例如:
```
@Service
public class UserServiceImpl implements UserService {
private static final String REDIS_KEY_PREFIX = "user:";
@Autowired
private UserMapper userMapper;
@Autowired
private RedisTemplate<String, Object> redisTemplate;
@Override
public User getUserById(Integer id) {
String key = REDIS_KEY_PREFIX + id;
User user = (User) redisTemplate.opsForValue().get(key);
if (user == null) {
user = userMapper.getUserById(id);
if (user != null) {
redisTemplate.opsForValue().set(key, user);
}
}
return user;
}
}
```
以上就是SpringBoot整合Mybatis和Redis的原理及实现步骤。
阅读全文
相关推荐
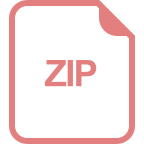
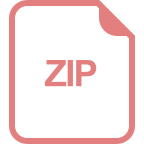

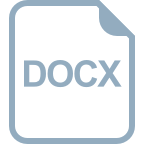
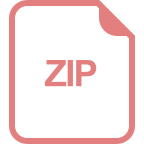
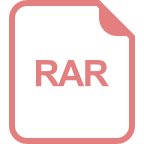
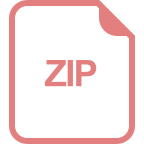
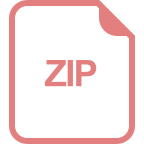
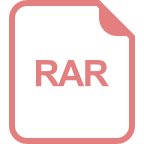
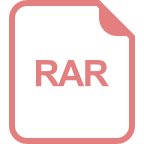
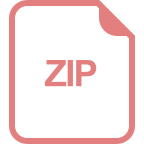
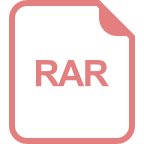
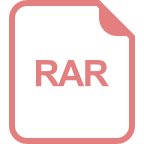
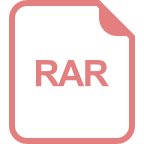
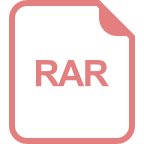
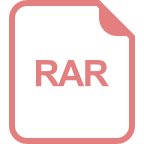
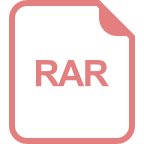
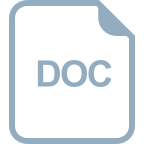