给出来 Python 代码: #include <bits/stdc++.h> using namespace std; int main() { int t; cin >> t; while(t--) { string s; cin >> s; int n = s.size(); set<char> st; if (n % 2) { for (int i = 0; i < n / 2; ++i) { st.insert(s[i]); } } else { for (int i = 0; i < n / 2 + 1; ++i) { st.insert(s[i]); } } cout << (st.size() != 1 ? "YES": "NO") << "\n"; } return 0; }
时间: 2024-01-13 19:04:59 浏览: 28
这段代码是 C++ 的,不是 Python 的,它实现了一个题目的解法。该题目是给定一个字符串,判断它能否通过删除一个字符后变为回文串。给定的代码中,使用了 set 容器来记录字符串中不同的字符,然后根据字符串长度是偶数还是奇数的不同情况,统计了需要考虑的字符数量。最后,判断不同字符的数量是否为 1,即可判断该字符串是否能够通过删除一个字符变为回文串。
以下是 Python 版本的代码:
```python
t = int(input())
for _ in range(t):
s = input().strip()
n = len(s)
st = set(s[:n//2] if n % 2 else s[:n//2+1])
print("YES" if len(st) != 1 else "NO")
```
这段代码与 C++ 版本实现的思路基本相同,使用了 Python 中的 set 容器来记录字符串中不同的字符,然后根据字符串长度的奇偶性,统计需要考虑的字符数量。最后,判断不同字符的数量是否为 1,即可判断该字符串是否能够通过删除一个字符变为回文串。
相关问题
请修改这段代码:#include<bits/stdc++.h> #include<cstring> #include<sstream> using namespace std; bool cmp(string x,string y) { return atoi(x.c_str())>atoi(y.c_str()); } int main() { int n; cin>>n; string str=to_string(n); sort(str.begin(), str.end(), cmp); cout<<stoi(str); return 0; }
以下是修改后的代码:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
bool cmp(char x, char y)
{
return x > y;
}
int main()
{
int n;
cin >> n;
string str = to_string(n);
vector<char> digits(str.begin(), str.end());
sort(digits.begin(), digits.end(), cmp);
string sortedStr(digits.begin(), digits.end());
int sortedNum = stoi(sortedStr);
cout << sortedNum;
return 0;
}
```
修改说明:
1. 移除了无关的头文件 `<bits/stdc++.h>` 和 `<cstring>`。
2. 添加了头文件 `<iostream>` 和 `<vector>`。
3. 修改了比较函数 `cmp`,将其参数由 `string` 类型改为 `char` 类型,以便在排序时直接对字符进行比较。
4. 使用 `vector<char>` 来保存整数的各个位上的数字。
5. 将排序后的字符数组转换为字符串 `sortedStr`。
6. 使用 `stoi` 将排序后的字符串转换为整数 `sortedNum`。
7. 输出排序后的整数。
请注意,这只是对原始代码的修改,不包含输入验证和错误处理。在实际使用中,应该添加适当的输入验证和错误处理来提高程序的健壮性。
#include<bits/stdc++.h> #include<cstring> #include<sstream> using namespace std; bool cmp(string x,string y) { return atoi(x.c_str())>atoi(y.c_str()); } int main() { int n; cin>>n; string str=to_string(n); sort(str.begin(), str.end(), cmp); cout<<stoi(str); return 0; }
这段代码是一个简单的整数排序程序,它将输入的整数按照降序排列后输出。以下是对代码的解释:
1. 头文件部分:
```cpp
#include<bits/stdc++.h>
#include<cstring>
#include<sstream>
```
这部分包含了一些需要使用的标准库和头文件。
2. 比较函数:
```cpp
bool cmp(string x,string y)
{
return atoi(x.c_str())>atoi(y.c_str());
}
```
这是一个自定义的比较函数,用于在排序时判断两个字符串代表的整数的大小。它将字符串转换为整数后进行比较。
3. 主函数:
```cpp
int main()
{
int n;
cin>>n;
string str=to_string(n);
sort(str.begin(), str.end(), cmp);
cout<<stoi(str);
return 0;
}
```
在主函数中,首先从标准输入读取一个整数n。然后,使用`to_string`将整数n转换为字符串str。
接下来,使用`sort`函数对字符串str进行排序,排序时使用了自定义的比较函数cmp。
最后,使用`stoi`函数将排序后的字符串str转换为整数,并将结果输出到标准输出。
请注意,这段代码可能存在一些问题,例如没有对输入进行验证和错误处理。在实际使用中,应该进行适当的输入验证和错误处理来确保程序的健壮性。
相关推荐
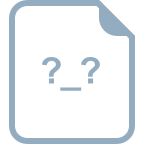
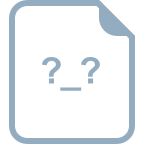
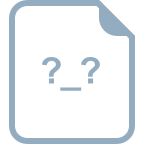


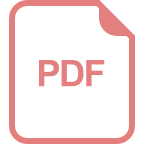
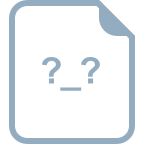
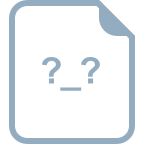
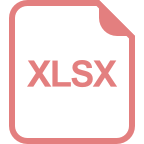
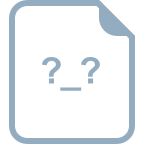
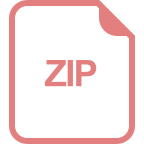