springboot配置ldaps,为什么需要同时导入ad根证书和ldaps证书,应该如何springboot应该如何导入,以及解决java不信任自签名的问题
时间: 2023-03-23 16:02:19 浏览: 490
在 Spring Boot 应用中配置 LDAP 和 LDAPS 权威服务器(Active Directory)认证,需要使用 LDAP 的 Java API,例如 JNDI(Java Naming and Directory Interface)。您需要在应用程序的 pom.xml 文件中添加以下依赖项:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-ldap</artifactId>
</dependency>
```
要使用 LDAPS,请确保您的 Active Directory 服务器已启用 LDAPS,并将 LDAPS URL 配置为类似于以下内容的格式:
```
ldaps://your.domain.com:636
```
您还需要为 Active Directory 根证书颁发机构创建一个 Java KeyStore,并将其添加到您的 Spring Boot 应用程序中。您可以使用 Java 的 keytool 命令生成 KeyStore:
```
keytool -importcert -keystore truststore.jks -file ad-root-ca.cer -alias ad-root-ca
```
请将证书替换为您的 Active Directory 根证书颁发机构的证书。
然后,在您的 Spring Boot 应用程序配置文件中,您需要指定以下属性:
```
spring.ldap.urls=ldaps://your.domain.com:636
spring.ldap.base=dc=your,dc=domain,dc=com
spring.ldap.username=your-username@your.domain.com
spring.ldap.password=your-password
spring.ldap.base-environment.javax.net.ssl.trust-store=classpath:truststore.jks
spring.ldap.base-environment.javax.net.ssl.trust-store-password=your-trust-store-password
```
请将这些属性替换为您的 Active Directory 配置值。此外,确保您的 Java 运行时版本支持 TLS 1.2,这是 LDAPS 所需的协议版本。
至于 Java 不信任自签名证书的问题,您可以将证书添加到 Java KeyStore 中,并在 Spring Boot 应用程序配置文件中使用上述方法指定 KeyStore。或者,您可以将以下属性添加到应用程序配置文件中,以信任所有自签名证书:
```
javax.net.ssl.trustStore=NONE
javax.net.ssl.trustStoreType=JKS
javax.net.ssl.trustStoreProvider=PKIX
javax.net.ssl.trustStorePassword=changeit
javax.net.ssl.keyStore=NONE
javax.net.ssl.keyStoreType=JKS
javax.net.ssl.keyStoreProvider=SunJSSE
javax.net.ssl.keyStorePassword=changeit
javax.net.ssl.trustAll=true
```
请注意,这样做可能会使您的应用程序容易受到安全漏洞的攻击,因此不建议在生产环境中使用。最好的解决方案是使用由受信任的证书颁发机构颁发的证书。
阅读全文
相关推荐
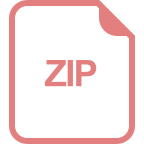



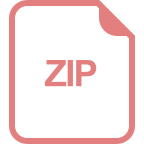
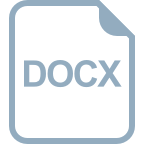
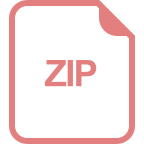
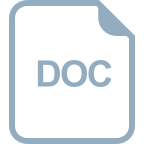








