javaweb网上蛋糕商城的商品分类排序模块代码
时间: 2023-11-22 10:23:18 浏览: 111
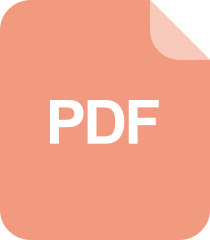
Javaweb项目实战:油画商城。第三集—-分类模块的实现!!!GOGOGOGO!!疫情还在,别乱跑呢,学习别停!!!
商品分类排序是电商平台常见的功能,可以让用户更方便地浏览和筛选商品。下面给出一个简单的示例代码:
1. 商品分类排序页面:
```html
<!-- 商品分类排序页面 -->
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>商品分类排序</title>
</head>
<body>
<!-- 商品分类列表 -->
<ul>
<li><a href="category.jsp?category=1">分类1</a></li>
<li><a href="category.jsp?category=2">分类2</a></li>
<li><a href="category.jsp?category=3">分类3</a></li>
</ul>
<!-- 商品排序方式 -->
<form action="category.jsp" method="get">
<input type="hidden" name="category" value="${category}">
<select name="sort">
<option value="price_asc">价格从低到高</option>
<option value="price_desc">价格从高到低</option>
<option value="sales_asc">销量从低到高</option>
<option value="sales_desc">销量从高到低</option>
</select>
<input type="submit" value="排序">
</form>
<!-- 商品列表 -->
<table>
<thead>
<tr>
<th>商品名称</th>
<th>价格</th>
<th>销量</th>
</tr>
</thead>
<tbody>
<!-- 根据分类和排序方式查询商品列表,并进行展示 -->
<%-- 代码略 --%>
</tbody>
</table>
</body>
</html>
```
2. 商品分类排序处理:
```java
// 商品分类列表页面
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 获取分类和排序方式参数
int category = Integer.parseInt(request.getParameter("category"));
String sort = request.getParameter("sort");
// 根据分类和排序方式查询商品列表
ProductService productService = new ProductService();
List<Product> productList = productService.getProductList(category, sort);
// 将商品列表存储到 request 中
request.setAttribute("productList", productList);
// 跳转到商品分类排序页面
request.getRequestDispatcher("category.jsp").forward(request, response);
}
```
3. 商品分类排序查询:
```java
// 根据分类和排序方式查询商品列表
public List<Product> getProductList(int category, String sort) {
// 构造 SQL 查询语句
String sql = "SELECT * FROM product WHERE category=?";
if (sort.equals("price_asc")) {
sql += " ORDER BY price ASC";
} else if (sort.equals("price_desc")) {
sql += " ORDER BY price DESC";
} else if (sort.equals("sales_asc")) {
sql += " ORDER BY sales ASC";
} else if (sort.equals("sales_desc")) {
sql += " ORDER BY sales DESC";
}
// 执行 SQL 查询,并将结果转换为 Product 对象列表
List<Product> productList = new ArrayList<Product>();
try (Connection conn = DbUtil.getConnection();
PreparedStatement stmt = conn.prepareStatement(sql)) {
stmt.setInt(1, category);
ResultSet rs = stmt.executeQuery();
while (rs.next()) {
Product product = new Product();
product.setId(rs.getInt("id"));
product.setName(rs.getString("name"));
product.setPrice(rs.getDouble("price"));
product.setSales(rs.getInt("sales"));
// 其他字段赋值
productList.add(product);
}
} catch (SQLException e) {
e.printStackTrace();
}
return productList;
}
```
以上代码仅供参考,具体实现方式可以根据项目需求进行调整。
阅读全文
相关推荐
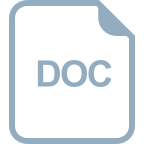
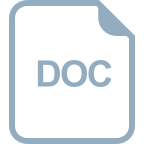
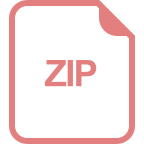
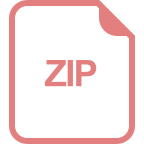
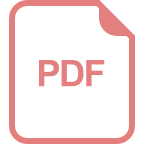
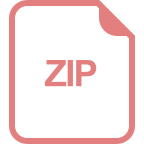
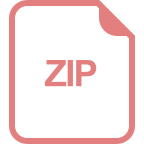
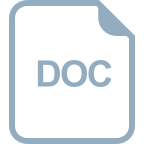
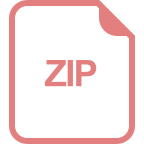
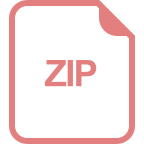
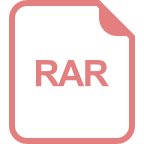
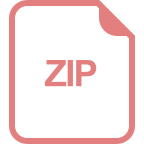
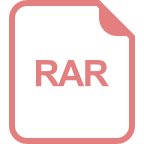
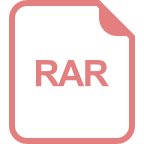
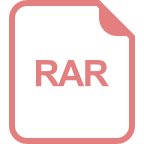
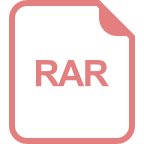
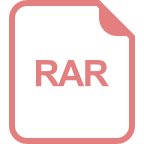
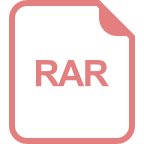