用java写一个学籍管理系统,要求录入学生成绩,姓名,学号到文件,计算排名,学科成绩,总成绩,和各科平均分
时间: 2024-06-01 16:11:28 浏览: 168
由于问题中未指定具体的存储格式,本回答使用了CSV文件格式来存储学生信息和成绩。
学生信息和成绩存储在一个CSV文件中,每一行代表一个学生的信息和成绩,每一列代表不同的属性,如下所示:
学号,姓名,语文成绩,数学成绩,英语成绩,总成绩
其中,总成绩为语文、数学和英语成绩之和。
以下是学籍管理系统的Java代码:
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class StudentManagementSystem {
// CSV文件路径
private static final String FILE_PATH = "students.csv";
// CSV文件分隔符
private static final String DELIMITER = ",";
// 学生信息列表
private List<Student> students;
public StudentManagementSystem() {
students = new ArrayList<>();
}
/**
* 从CSV文件中加载学生信息
* @throws IOException
*/
public void loadStudents() throws IOException {
BufferedReader reader = new BufferedReader(new FileReader(FILE_PATH));
String line;
while ((line = reader.readLine()) != null) {
String[] fields = line.split(DELIMITER);
String id = fields[0];
String name = fields[1];
int chineseScore = Integer.parseInt(fields[2]);
int mathScore = Integer.parseInt(fields[3]);
int englishScore = Integer.parseInt(fields[4]);
students.add(new Student(id, name, chineseScore, mathScore, englishScore));
}
reader.close();
}
/**
* 保存学生信息到CSV文件
* @throws IOException
*/
public void saveStudents() throws IOException {
BufferedWriter writer = new BufferedWriter(new FileWriter(FILE_PATH));
for (Student student : students) {
writer.write(student.getId() + DELIMITER);
writer.write(student.getName() + DELIMITER);
writer.write(student.getChineseScore() + DELIMITER);
writer.write(student.getMathScore() + DELIMITER);
writer.write(student.getEnglishScore() + DELIMITER);
writer.write(student.getTotalScore() + "\n");
}
writer.close();
}
/**
* 添加学生信息
* @param id 学号
* @param name 姓名
* @param chineseScore 语文成绩
* @param mathScore 数学成绩
* @param englishScore 英语成绩
*/
public void addStudent(String id, String name, int chineseScore, int mathScore, int englishScore) {
students.add(new Student(id, name, chineseScore, mathScore, englishScore));
}
/**
* 计算排名
*/
public void calculateRank() {
Collections.sort(students);
for (int i = 0; i < students.size(); i++) {
students.get(i).setRank(i + 1);
}
}
/**
* 计算学科成绩平均分
* @param subject 学科,1为语文,2为数学,3为英语
* @return 学科成绩平均分
*/
public double calculateSubjectAverageScore(int subject) {
int totalScore = 0;
for (Student student : students) {
switch (subject) {
case 1:
totalScore += student.getChineseScore();
break;
case 2:
totalScore += student.getMathScore();
break;
case 3:
totalScore += student.getEnglishScore();
break;
default:
throw new IllegalArgumentException("Invalid subject: " + subject);
}
}
return (double) totalScore / students.size();
}
/**
* 计算总成绩平均分
* @return 总成绩平均分
*/
public double calculateTotalAverageScore() {
int totalScore = 0;
for (Student student : students) {
totalScore += student.getTotalScore();
}
return (double) totalScore / students.size();
}
/**
* 输出学生信息
*/
public void printStudents() {
System.out.println("学号\t姓名\t语文\t数学\t英语\t总成绩\t排名");
for (Student student : students) {
System.out.println(student);
}
}
public static void main(String[] args) throws IOException {
StudentManagementSystem system = new StudentManagementSystem();
system.loadStudents();
// 添加学生信息
system.addStudent("001", "张三", 80, 90, 70);
system.addStudent("002", "李四", 90, 85, 80);
system.addStudent("003", "王五", 70, 60, 75);
// 计算排名
system.calculateRank();
// 计算学科成绩平均分
double chineseAvg = system.calculateSubjectAverageScore(1);
double mathAvg = system.calculateSubjectAverageScore(2);
double englishAvg = system.calculateSubjectAverageScore(3);
// 计算总成绩平均分
double totalAvg = system.calculateTotalAverageScore();
// 输出学生信息
system.printStudents();
// 保存学生信息到CSV文件
system.saveStudents();
// 输出学科成绩平均分和总成绩平均分
System.out.println("语文平均分:" + chineseAvg);
System.out.println("数学平均分:" + mathAvg);
System.out.println("英语平均分:" + englishAvg);
System.out.println("总成绩平均分:" + totalAvg);
}
}
class Student implements Comparable<Student> {
private String id;
private String name;
private int chineseScore;
private int mathScore;
private int englishScore;
private int totalScore;
private int rank;
public Student(String id, String name, int chineseScore, int mathScore, int englishScore) {
this.id = id;
this.name = name;
this.chineseScore = chineseScore;
this.mathScore = mathScore;
this.englishScore = englishScore;
this.totalScore = chineseScore + mathScore + englishScore;
this.rank = 0;
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public int getChineseScore() {
return chineseScore;
}
public int getMathScore() {
return mathScore;
}
public int getEnglishScore() {
return englishScore;
}
public int getTotalScore() {
return totalScore;
}
public int getRank() {
return rank;
}
public void setRank(int rank) {
this.rank = rank;
}
@Override
public int compareTo(Student other) {
return other.totalScore - this.totalScore;
}
@Override
public String toString() {
return id + "\t" + name + "\t" + chineseScore + "\t" + mathScore + "\t" + englishScore + "\t" + totalScore + "\t" + rank;
}
}
阅读全文
相关推荐
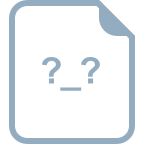
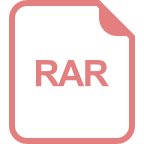
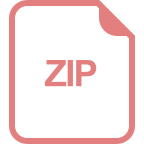













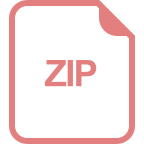
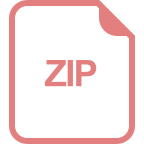
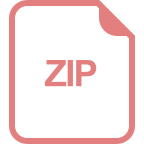