用vc++6.0做的屏幕截取程序(含代码)
时间: 2024-03-02 22:53:59 浏览: 128
以下是一个简单的用 VC++ 6.0 实现屏幕截取的程序:
```c++
#include <windows.h>
#include <iostream.h>
int main()
{
// 获取屏幕DC
HDC hScreenDC = GetDC(NULL);
// 获取屏幕尺寸
int nScreenWidth = GetSystemMetrics(SM_CXSCREEN);
int nScreenHeight = GetSystemMetrics(SM_CYSCREEN);
// 创建内存DC
HDC hMemoryDC = CreateCompatibleDC(hScreenDC);
// 创建位图对象
HBITMAP hBitmap = CreateCompatibleBitmap(hScreenDC, nScreenWidth, nScreenHeight);
// 将位图选入内存DC
SelectObject(hMemoryDC, hBitmap);
// 将屏幕内容复制到内存DC中
BitBlt(hMemoryDC, 0, 0, nScreenWidth, nScreenHeight, hScreenDC, 0, 0, SRCCOPY);
// 将位图保存为文件
HANDLE hFile = CreateFile("screenshot.bmp", GENERIC_WRITE, 0, NULL, CREATE_ALWAYS, FILE_ATTRIBUTE_NORMAL, NULL);
if (hFile == INVALID_HANDLE_VALUE) {
cout << "Failed to create file" << endl;
return 1;
}
BITMAPFILEHEADER bfh = { 0 };
bfh.bfType = 0x4d42;
bfh.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + nScreenWidth * nScreenHeight * 4;
bfh.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER);
DWORD dwBytesWritten;
WriteFile(hFile, &bfh, sizeof(BITMAPFILEHEADER), &dwBytesWritten, NULL);
BITMAPINFOHEADER bih = { 0 };
bih.biSize = sizeof(BITMAPINFOHEADER);
bih.biWidth = nScreenWidth;
bih.biHeight = nScreenHeight;
bih.biPlanes = 1;
bih.biBitCount = 32;
WriteFile(hFile, &bih, sizeof(BITMAPINFOHEADER), &dwBytesWritten, NULL);
BYTE* pBits = new BYTE[nScreenWidth * nScreenHeight * 4];
GetBitmapBits(hBitmap, nScreenWidth * nScreenHeight * 4, pBits);
WriteFile(hFile, pBits, nScreenWidth * nScreenHeight * 4, &dwBytesWritten, NULL);
CloseHandle(hFile);
delete[] pBits;
// 释放资源
DeleteObject(hBitmap);
DeleteDC(hMemoryDC);
ReleaseDC(NULL, hScreenDC);
return 0;
}
```
该程序使用了 Win32 API 的相关函数,通过获取屏幕设备上下文(DC),将屏幕内容复制到内存 DC 中,然后将内存 DC 中的位图保存为文件。请注意,此程序只支持保存为 BMP 格式的位图,如果需要支持其他格式,需要使用相应的 API 函数。
阅读全文
相关推荐
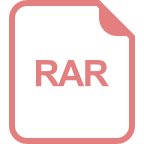
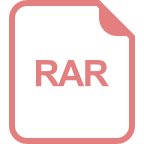
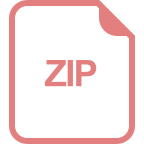
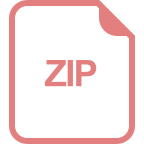
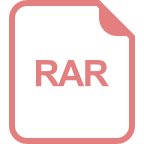
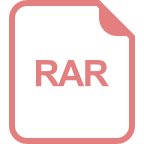
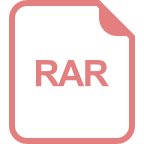
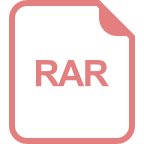
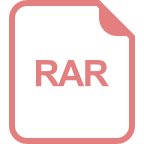
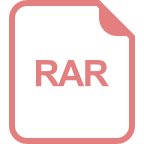
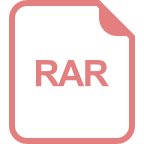
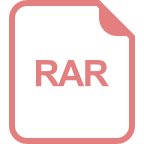