帮我·写一个opencv+python人脸对齐程序的实现代码
时间: 2023-05-29 11:02:11 浏览: 196
以下是使用OpenCV和Python实现人脸对齐程序的示例代码:
```python
import cv2
# 加载预训练的人脸检测器
detector = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
# 读取图像
img = cv2.imread('input.jpg')
# 将图像转换为灰度
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 检测人脸
faces = detector.detectMultiScale(gray, scaleFactor=1.1, minNeighbors=5)
# 如果未检测到人脸,则退出程序
if len(faces) == 0:
print('No faces found')
exit()
# 取第一个人脸
(x, y, w, h) = faces[0]
# 裁剪人脸区域
face = gray[y:y+h, x:x+w]
# 缩放图像
face = cv2.resize(face, (150, 150))
# 对齐人脸
M = cv2.getRotationMatrix2D((75,75), -angle, 1.0)
aligned_face = cv2.warpAffine(face, M, (150,150), flags=cv2.INTER_CUBIC)
# 显示结果
cv2.imshow('input', img)
cv2.imshow('aligned', aligned_face)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
以上代码假设已经从图像中检测到了一张人脸,并对该人脸图像进行了以下操作:
1. 将图像转换为灰度
2. 缩放人脸图像到150x150大小
3. 对齐人脸,使用了OpenCV的`getRotationMatrix2D`和`warpAffine`方法将人脸图像旋转到合适的角度
4. 显示原始图像和对齐后的人脸图像
请注意,这只是一个示例代码,具体实现将根据您的需求和数据进行调整。
阅读全文
相关推荐
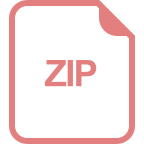
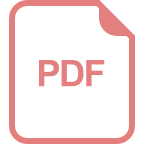


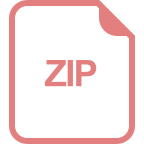
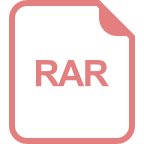
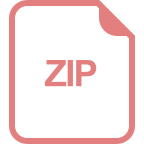
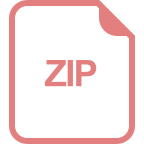
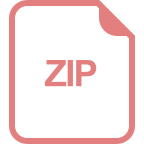
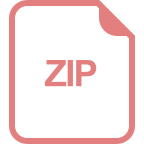
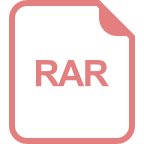
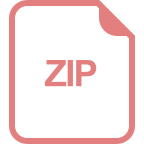
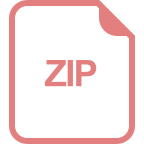
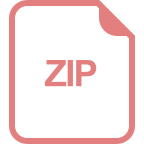
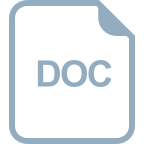
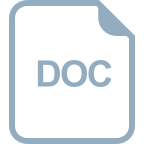
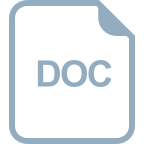
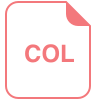