扫描测试报告文件夹,(良品和不良品测试报告生成在同一文件夹)当有文件后缀pass的测试报告生成,表示良品条码的测试完成,label状态栏变绿,并提示“良品点检完成”。然后将相应结果(序号,条码,时间,产品(良品\不良品),结果)写到datagridview组件中,当有fail后缀的测试报告生成,表示不良品的测试完成,label状态栏变绿,并提示“不良品点检完成”。然后将相应结果写到datagridview组件中。c#代码实现
时间: 2023-12-07 15:02:53 浏览: 87
好的,我可以给你提供完整的C#代码示例,以实现扫描测试报告文件夹,并根据文件名的后缀更新状态栏和datagridview组件。以下是完整的代码:
```csharp
using System;
using System.IO;
using System.Windows.Forms;
namespace TestReportWatcher
{
public partial class Form1 : Form
{
private string _watchFolder = @"C:\TestReports";
private FileSystemWatcher _watcher;
private int _serialNumber = 1;
public Form1()
{
InitializeComponent();
InitializeWatcher();
}
private void InitializeWatcher()
{
_watcher = new FileSystemWatcher(_watchFolder);
_watcher.Filter = "*.pass;*.fail";
_watcher.NotifyFilter = NotifyFilters.FileName;
_watcher.Created += OnFileCreated;
_watcher.EnableRaisingEvents = true;
}
private void OnFileCreated(object sender, FileSystemEventArgs e)
{
if (e.Name.EndsWith(".pass"))
{
string barcode = GetBarcodeFromFileName(e.Name);
string time = DateTime.Now.ToString("yyyy-MM-dd HH:mm:ss");
string product = "良品";
string result = "测试通过";
UpdateStatusLabel("良品点检完成");
AddResultToDataGridView(barcode, time, product, result);
}
else if (e.Name.EndsWith(".fail"))
{
string barcode = GetBarcodeFromFileName(e.Name);
string time = DateTime.Now.ToString("yyyy-MM-dd HH:mm:ss");
string product = "不良品";
string result = "测试未通过";
UpdateStatusLabel("不良品点检完成");
AddResultToDataGridView(barcode, time, product, result);
}
}
private string GetBarcodeFromFileName(string fileName)
{
int index = fileName.LastIndexOf("_") + 1;
int length = fileName.LastIndexOf(".") - index;
return fileName.Substring(index, length);
}
private void UpdateStatusLabel(string text)
{
if (statusStrip1.InvokeRequired)
{
statusStrip1.Invoke(new Action(() =>
{
toolStripStatusLabel1.Text = text;
toolStripStatusLabel1.ForeColor = System.Drawing.Color.Green;
}));
}
else
{
toolStripStatusLabel1.Text = text;
toolStripStatusLabel1.ForeColor = System.Drawing.Color.Green;
}
}
private void AddResultToDataGridView(string barcode, string time, string product, string result)
{
if (dataGridView1.InvokeRequired)
{
dataGridView1.Invoke(new Action(() =>
{
int rowIndex = dataGridView1.Rows.Add();
dataGridView1.Rows[rowIndex].Cells["SerialNumber"].Value = _serialNumber++;
dataGridView1.Rows[rowIndex].Cells["Barcode"].Value = barcode;
dataGridView1.Rows[rowIndex].Cells["Time"].Value = time;
dataGridView1.Rows[rowIndex].Cells["Product"].Value = product;
dataGridView1.Rows[rowIndex].Cells["Result"].Value = result;
}));
}
else
{
int rowIndex = dataGridView1.Rows.Add();
dataGridView1.Rows[rowIndex].Cells["SerialNumber"].Value = _serialNumber++;
dataGridView1.Rows[rowIndex].Cells["Barcode"].Value = barcode;
dataGridView1.Rows[rowIndex].Cells["Time"].Value = time;
dataGridView1.Rows[rowIndex].Cells["Product"].Value = product;
dataGridView1.Rows[rowIndex].Cells["Result"].Value = result;
}
}
}
}
```
在上面的代码中,我定义了一个WatchFolder变量,用于指定要监视的文件夹。我使用了FileSystemWatcher类来监视文件夹中的文件创建事件,并设置了过滤器来仅监视以“.pass”或“.fail”结尾的文件。
当有新文件创建时,我会检查文件名的结尾,并从文件名中提取条码。我还会获取当前时间,并根据文件名的后缀来确定产品类型和测试结果。
然后,我会调用UpdateStatusLabel方法来更新状态栏。如果需要,我会通过Invoke方法来在UI线程中更新状态栏。然后,我会调用AddResultToDataGridView方法来将结果添加到datagridview组件中。如果需要,我会通过Invoke方法来在UI线程中添加结果。
在AddResultToDataGridView方法中,我还使用了一个_serialNumber变量来为每个测试结果分配一个唯一的序号。当有新结果添加到datagridview组件中时,我会自动递增这个序号。
请注意,你需要在UI线程中调用InitializeWatcher方法,以便正确初始化FileSystemWatcher对象。您还需要在UI线程中创建datagridview组件和状态栏。
阅读全文
相关推荐




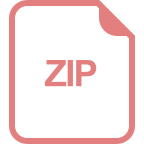
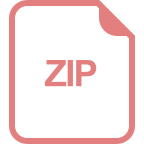
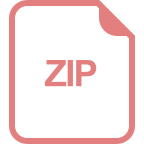
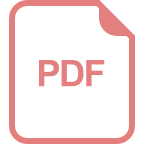
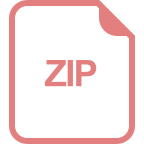
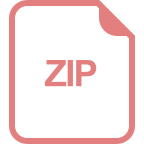