有 5 个文件分别是 main.c、visit.h、study.h、 visit.c、study.c,如果上述 5 个文件在同一目录下,请编写 Makefile 文件
时间: 2024-03-23 12:39:09 浏览: 79
好的,以下是 Makefile 文件的编写:
```makefile
CC = gcc
CFLAGS = -Wall -Wextra
SRC = main.c visit.c study.c
OBJ = $(SRC:.c=.o)
DEPS = visit.h study.h
all: program
program: $(OBJ)
$(CC) $(CFLAGS) $^ -o $@
%.o: %.c $(DEPS)
$(CC) $(CFLAGS) -c $<
clean:
rm -f $(OBJ) program
```
这个 Makefile 文件中包含了以下几个目标:
- `all`:默认的构建目标,它依赖于 `program` 目标;
- `program`:生成可执行文件 `program` 的目标;
- `clean`:清除编译产生的中间文件和可执行文件的目标。
其中,变量的含义如下:
- `CC`:编译器;
- `CFLAGS`:编译选项;
- `SRC`:源文件列表;
- `OBJ`:目标文件列表,它是通过将每个源文件的后缀名从 `.c` 改为 `.o` 得到的;
- `DEPS`:头文件列表。
这个 Makefile 文件中的规则如下:
- `all` 目标依赖于 `program` 目标;
- `program` 目标依赖于 `$(OBJ)` 列表中的所有目标文件;
- 每个目标文件都是通过对应的源文件和 `$(DEPS)` 中的头文件生成的;
- `clean` 目标只是简单地删除中间文件和可执行文件。
阅读全文
相关推荐
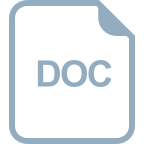
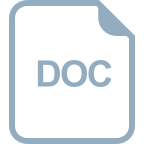
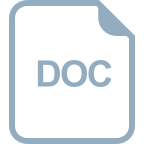
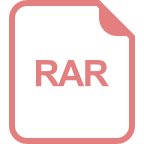
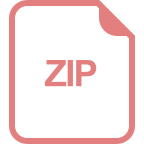
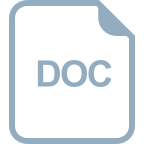
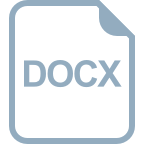
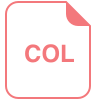
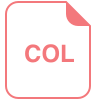
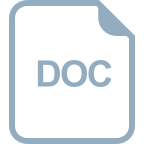
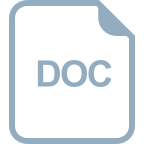
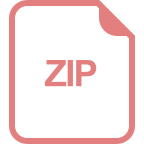
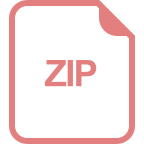
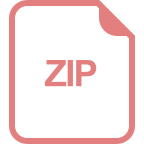
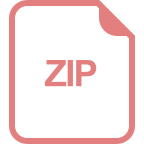
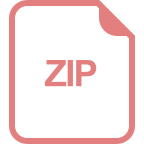