用arduino实现土壤湿度检测并把湿度数据通过WIFI上传到blinker app
时间: 2024-02-03 17:12:02 浏览: 90
实现这个功能,需要以下步骤:
1. 购买土壤湿度传感器和ESP8266 WIFI模块。
2. 将土壤湿度传感器连接到Arduino的模拟输入引脚。
3. 将ESP8266 WIFI模块连接到Arduino的数字引脚,并使用软串口库将它与Arduino通信。
4. 在Arduino代码中,读取土壤湿度传感器的值,并将其转换为百分比。
5. 将湿度数据通过WIFI上传到Blinker App。可以使用Blinker库来实现这一点。
下面是一个简单的代码示例:
```
#include <SoftwareSerial.h>
#include <Blinker.h>
// Define the pins for the soil moisture sensor
#define SOIL_MOISTURE_SENSOR_PIN A0
// Define the pins for the ESP8266 WIFI module
#define RX_PIN 2
#define TX_PIN 3
// Define the WIFI credentials
#define WIFI_SSID "your_wifi_ssid"
#define WIFI_PASSWORD "your_wifi_password"
// Define the Blinker credentials
#define BLINKER_AUTHKEY "your_blinker_authkey"
#define BLINKER_DEVID "your_blinker_devid"
SoftwareSerial esp8266(RX_PIN, TX_PIN);
BlinkerButton btn = BlinkerButton("btn");
void setup() {
Serial.begin(9600);
// Initialize the soil moisture sensor pin
pinMode(SOIL_MOISTURE_SENSOR_PIN, INPUT);
// Initialize the ESP8266 WIFI module
esp8266.begin(9600);
esp8266.println("AT+RST");
delay(1000);
esp8266.println("AT+CWMODE=1");
esp8266.println("AT+CWJAP=\"" WIFI_SSID "\",\"" WIFI_PASSWORD "\"");
delay(5000);
// Initialize the Blinker library
Blinker.begin(BLINKER_AUTHKEY, BLINKER_DEVID);
Blinker.attachData("soil_moisture", getSoilMoisture);
}
void loop() {
Blinker.run();
}
int getSoilMoisture() {
// Read the soil moisture sensor value and convert to percentage
int sensorValue = analogRead(SOIL_MOISTURE_SENSOR_PIN);
int percentage = map(sensorValue, 0, 1023, 0, 100);
// Send the percentage value to Blinker
return percentage;
}
```
在Blinker App中,创建一个新的设备,并将其与代码中定义的BLINKER_DEVID匹配。然后,将代码上传到Arduino板上,打开串口监视器,确认WIFI连接成功,并检查数据是否已成功上传到Blinker App。
阅读全文
相关推荐
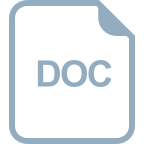
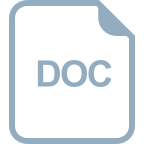
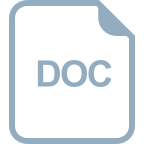


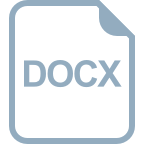


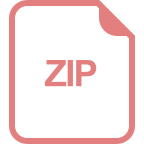









