crow::json::rvalue源代码
时间: 2023-07-30 08:10:45 浏览: 70
以下是Crow库中的`crow::json::rvalue`类的源代码:
```c++
namespace crow {
namespace json {
class rvalue {
enum class data_type {
NUL,
NUMBER,
STRING,
BOOL,
OBJECT,
ARRAY
};
data_type type_;
union {
double number_;
std::string* string_;
bool bool_;
object* object_;
array* array_;
};
public:
rvalue() : type_(data_type::NUL) {}
rvalue(std::nullptr_t) : type_(data_type::NUL) {}
rvalue(double val) : type_(data_type::NUMBER), number_(val) {}
rvalue(const std::string& val) : type_(data_type::STRING), string_(new std::string(val)) {}
rvalue(std::string&& val) : type_(data_type::STRING), string_(new std::string(std::move(val))) {}
rvalue(bool val) : type_(data_type::BOOL), bool_(val) {}
rvalue(object&& val) : type_(data_type::OBJECT), object_(new object(std::move(val))) {}
rvalue(array&& val) : type_(data_type::ARRAY), array_(new array(std::move(val))) {}
rvalue(const object& val) : type_(data_type::OBJECT), object_(new object(val)) {}
rvalue(const array& val) : type_(data_type::ARRAY), array_(new array(val)) {}
rvalue(rvalue&& other) noexcept {
type_ = other.type_;
switch (type_) {
case data_type::NUL:
break;
case data_type::NUMBER:
number_ = other.number_;
break;
case data_type::BOOL:
bool_ = other.bool_;
break;
case data_type::STRING:
string_ = other.string_;
break;
case data_type::OBJECT:
object_ = other.object_;
break;
case data_type::ARRAY:
array_ = other.array_;
break;
}
other.type_ = data_type::NUL;
}
rvalue(const rvalue& other) {
type_ = other.type_;
switch (type_) {
case data_type::NUL:
break;
case data_type::NUMBER:
number_ = other.number_;
break;
case data_type::BOOL:
bool_ = other.bool_;
break;
case data_type::STRING:
string_ = new std::string(*other.string_);
break;
case data_type::OBJECT:
object_ = new object(*other.object_);
break;
case data_type::ARRAY:
array_ = new array(*other.array_);
break;
}
}
rvalue& operator=(rvalue&& other) noexcept {
if (this != &other) {
type_ = other.type_;
switch (type_) {
case data_type::NUL:
break;
case data_type::NUMBER:
number_ = other.number_;
break;
case data_type::BOOL:
bool_ = other.bool_;
break;
case data_type::STRING:
string_ = other.string_;
break;
case data_type::OBJECT:
object_ = other.object_;
break;
case data_type::ARRAY:
array_ = other.array_;
break;
}
other.type_ = data_type::NUL;
}
return *this;
}
rvalue& operator=(const rvalue& other) {
if (this != &other) {
type_ = other.type_;
switch (type_) {
case data_type::NUL:
break;
case data_type::NUMBER:
number_ = other.number_;
break;
case data_type::BOOL:
bool_ = other.bool_;
break;
case data_type::STRING:
string_ = new std::string(*other.string_);
break;
case data_type::OBJECT:
object_ = new object(*other.object_);
break;
case data_type::ARRAY:
array_ = new array(*other.array_);
break;
}
}
return *this;
}
~rvalue() {
switch (type_) {
case data_type::STRING:
delete string_;
break;
case data_type::OBJECT:
delete object_;
break;
case data_type::ARRAY:
delete array_;
break;
default:
break;
}
}
data_type type() const {
return type_;
}
double number() const {
return number_;
}
bool boolean() const {
return bool_;
}
const std::string& str() const {
return *string_;
}
const object& obj() const {
return *object_;
}
const array& arr() const {
return *array_;
}
};
}
}
```
`crow::json::rvalue`是一个C++类,用于表示JSON数据类型。其中,`data_type`是一个枚举类,表示JSON数据类型,包括空值、数字、字符串、布尔、对象和数组。`rvalue`类包括了默认构造函数、不同类型的构造函数、移动构造函数、拷贝构造函数、移动赋值运算符、拷贝赋值运算符和析构函数。它还包括了一些方法来获取不同类型的数据。
相关推荐
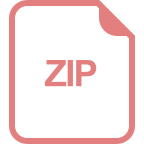
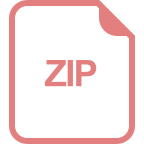
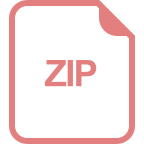














