给下面代码添加注释 public class TestJdbcTemplate { JdbcTemplate template; @Before public void init() throws Exception { template= new JdbcTemplate(DruidUtils.getDataSource()); } @Test public void testInsert() throws Exception { template.update("insert into account(name,balance) values(?,?)","王麻子",8888); } @Test public void testUpdate() throws Exception { template.update("update account set balance=? where id=?",4444,4); } @Test public void testDelete() throws Exception { template.update("delete from account where id=?",4); } @Test public void testFindAll() throws Exception { List<Emp1> emps = template.query("select * from emp", new BeanPropertyRowMapper<Emp1>(Emp1.class)); for (Emp1 e :emps) { System.out.println(e); } } @Test public void testFindCount(){ Integer count = template.queryForObject("select count(1) from emp", Integer.class); System.out.println(count); } @Test public void testFindByPage() throws Exception { List<Emp1> emps = template.query("select * from emp limit ?,?", new BeanPropertyRowMapper<Emp1>(Emp1.class),10,5); for (Emp1 e :emps) { System.out.println(e); } } }
时间: 2024-02-15 21:26:59 浏览: 70
// 导入JdbcTemplate类
public class TestJdbcTemplate {
// 声明一个JdbcTemplate对象
JdbcTemplate template;
// 在执行每个测试方法前初始化JdbcTemplate对象
@Before
public void init() throws Exception {
// 获取数据源并创建JdbcTemplate对象
template= new JdbcTemplate(DruidUtils.getDataSource());
}
// 测试插入操作
@Test
public void testInsert() throws Exception {
// 使用JdbcTemplate的update方法插入数据
template.update("insert into account(name,balance) values(?,?)","王麻子",8888);
}
// 测试更新操作
@Test
public void testUpdate() throws Exception {
// 使用JdbcTemplate的update方法更新数据
template.update("update account set balance=? where id=?",4444,4);
}
// 测试删除操作
@Test
public void testDelete() throws Exception {
// 使用JdbcTemplate的update方法删除数据
template.update("delete from account where id=?",4);
}
// 测试查询所有数据操作
@Test
public void testFindAll() throws Exception {
// 使用JdbcTemplate的query方法查询数据,并将结果转换为Emp1类的List
List<Emp1> emps = template.query("select * from emp", new BeanPropertyRowMapper<Emp1>(Emp1.class));
// 遍历List并输出每个Emp1对象
for (Emp1 e :emps) {
System.out.println(e);
}
}
// 测试查询数据条数操作
@Test
public void testFindCount(){
// 使用JdbcTemplate的queryForObject方法查询数据条数
Integer count = template.queryForObject("select count(1) from emp", Integer.class);
// 输出查询结果
System.out.println(count);
}
// 测试分页查询操作
@Test
public void testFindByPage() throws Exception {
// 使用JdbcTemplate的query方法分页查询数据,并将结果转换为Emp1类的List
List<Emp1> emps = template.query("select * from emp limit ?,?", new BeanPropertyRowMapper<Emp1>(Emp1.class),10,5);
// 遍历List并输出每个Emp1对象
for (Emp1 e :emps) {
System.out.println(e);
}
}
}
阅读全文
相关推荐
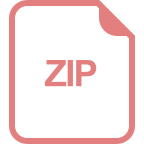
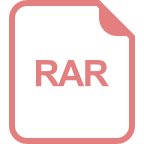
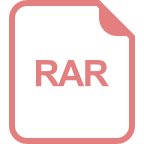
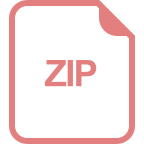
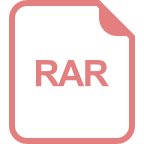
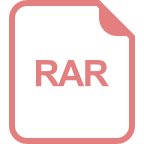
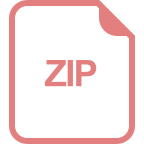
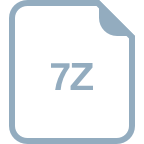
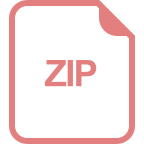
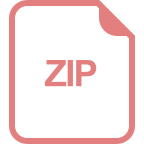
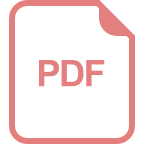
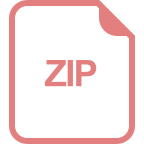
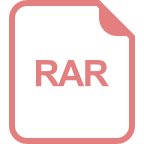
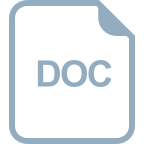



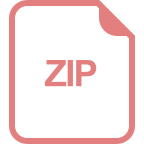