在javaScript中实现继承方法
时间: 2024-02-27 18:54:17 浏览: 21
在 JavaScript 中,实现继承方法有以下几种方式:
1. 原型继承:利用原型链实现继承。通过将父类的实例作为子类的原型,子类就可以访问到父类的属性和方法。例如:
```javascript
function Parent() {
this.name = 'parent';
}
Parent.prototype.sayName = function() {
console.log(this.name);
}
function Child() {}
Child.prototype = new Parent();
var child = new Child();
child.sayName(); // 输出:parent
```
2. 构造函数继承:利用 call 或 apply 方法将父类构造函数的作用域赋给子类。这种方式可以实现多继承。例如:
```javascript
function Parent(name) {
this.name = name;
}
function Child(name) {
Parent.call(this, name);
}
var child = new Child('child');
console.log(child.name); // 输出:child
```
3. 组合继承:结合原型继承和构造函数继承,既可以继承父类的属性和方法,又可以实现子类实例的独立。例如:
```javascript
function Parent(name) {
this.name = name;
}
Parent.prototype.sayName = function() {
console.log(this.name);
}
function Child(name, age) {
Parent.call(this, name);
this.age = age;
}
Child.prototype = new Parent();
Child.prototype.constructor = Child;
var child = new Child('child', 18);
console.log(child.name); // 输出:child
console.log(child.age); // 输出:18
child.sayName(); // 输出:child
```
4. 原型式继承:利用 Object.create() 方法创建一个新对象,以某个对象为原型,然后再对新对象进行修改。例如:
```javascript
var parent = {
name: 'parent',
sayName: function() {
console.log(this.name);
}
};
var child = Object.create(parent, {
name: {
value: 'child'
}
});
child.sayName(); // 输出:child
```
5. 寄生式继承:与原型式继承类似,但是在增强对象的方法时使用了一个函数封装。例如:
```javascript
var parent = {
name: 'parent',
sayName: function() {
console.log(this.name);
}
};
function createChild(original) {
var child = Object.create(original);
child.sayName = function() {
console.log('hello, ' + this.name);
}
return child;
}
var child = createChild(parent);
child.sayName(); // 输出:hello, parent
```
需要注意的是,在实现继承时应该注意避免出现属性和方法的重复定义,以及避免在父类的原型上修改引用类型的属性。
相关推荐
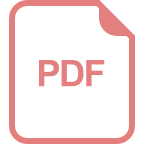














