Java自己写个线程池
时间: 2023-07-08 20:48:21 浏览: 47
自己实现一个简单的线程池,可以通过以下步骤完成:
1. 定义一个任务接口`Task`,用来表示需要执行的任务。
```
public interface Task {
void execute();
}
```
2. 定义一个工作线程类`WorkerThread`,用来执行任务。
```
public class WorkerThread extends Thread {
private final BlockingQueue<Task> taskQueue;
public WorkerThread(BlockingQueue<Task> taskQueue) {
this.taskQueue = taskQueue;
}
@Override
public void run() {
while (!Thread.currentThread().isInterrupted()) {
try {
Task task = taskQueue.take();
task.execute();
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
}
```
3. 定义一个线程池类`ThreadPool`,用来管理线程池。
```
public class ThreadPool {
private final int nThreads;
private final BlockingQueue<Task> taskQueue;
private final List<WorkerThread> threads = new ArrayList<>();
public ThreadPool(int nThreads, int queueSize) {
this.nThreads = nThreads;
taskQueue = new LinkedBlockingQueue<>(queueSize);
for (int i = 0; i < nThreads; i++) {
WorkerThread workerThread = new WorkerThread(taskQueue);
threads.add(workerThread);
workerThread.start();
}
}
public void execute(Task task) throws InterruptedException {
taskQueue.put(task);
}
public void shutdown() {
for (WorkerThread thread : threads) {
thread.interrupt();
}
}
}
```
在上面的代码中,我们使用`BlockingQueue`来存储任务队列,用`WorkerThread`来执行任务,使用`ThreadPool`来管理线程池。
4. 使用线程池来执行任务。
```
public class Main {
public static void main(String[] args) throws InterruptedException {
ThreadPool threadPool = new ThreadPool(5, 10);
for (int i = 0; i < 20; i++) {
int taskId = i;
threadPool.execute(new Task() {
@Override
public void execute() {
System.out.println("Task " + taskId + " is running on thread " + Thread.currentThread().getName());
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
});
}
threadPool.shutdown();
}
}
```
在上面的代码中,我们使用自己实现的线程池来执行20个任务,每个任务需要执行1秒钟,最后关闭线程池。
注意,这个自己实现的线程池只是一个简单的示例,没有考虑线程池的扩展性、性能等问题。在实际应用中,建议使用Java提供的线程池实现。
相关推荐
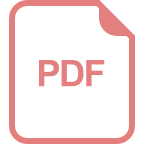
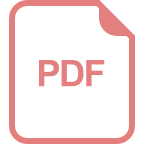
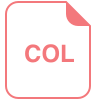
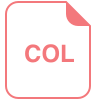
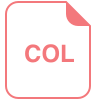
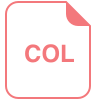
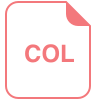









