Java怎么自动生成二维码
时间: 2023-12-10 07:03:59 浏览: 53
Java可以使用第三方库来生成二维码。其中比较常用的是ZXing(Zebra Crossing),它是一个开源的条码/二维码处理类库,支持多种编程语言,包括Java。
下面是一个示例代码,演示如何使用ZXing来生成二维码:
```java
import java.awt.Color;
import java.awt.Graphics2D;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import javax.imageio.ImageIO;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.WriterException;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.QRCodeWriter;
public class QrCodeGenerator {
public static void main(String[] args) throws IOException, WriterException {
String text = "https://www.example.com"; // 二维码内容
int width = 300; // 二维码宽度
int height = 300; // 二维码高度
String format = "png"; // 二维码格式
// 设置二维码参数
Map<EncodeHintType, Object> hints = new HashMap<>();
hints.put(EncodeHintType.CHARACTER_SET, "UTF-8");
// 生成二维码
QRCodeWriter qrCodeWriter = new QRCodeWriter();
BitMatrix bitMatrix = qrCodeWriter.encode(text, BarcodeFormat.QR_CODE, width, height, hints);
// 创建 BufferedImage 对象,并设置背景颜色和前景颜色
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
image.createGraphics();
Graphics2D graphics = (Graphics2D) image.getGraphics();
graphics.setColor(Color.WHITE);
graphics.fillRect(0, 0, width, height);
graphics.setColor(Color.BLACK);
// 绘制二维码
for (int i = 0; i < width; i++) {
for (int j = 0; j < height; j++) {
if (bitMatrix.get(i, j)) {
graphics.fillRect(i, j, 1, 1);
}
}
}
// 输出二维码到文件
File file = new File("qrcode." + format);
ImageIO.write(image, format, file);
}
}
```
运行该代码后,会在当前目录下生成一个名为“qrcode.png”的二维码图片。
相关推荐
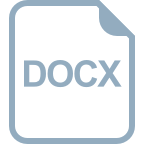
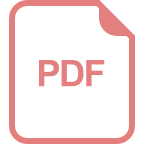














