vue3获取到页面元素
时间: 2023-09-15 22:20:03 浏览: 147
在Vue3中,可以使用 `ref` 来获取页面元素。具体步骤如下:
1. 在模板中使用 `ref`,为需要获取的元素添加一个标识符,例如:
```html
<template>
<div>
<input type="text" ref="myInput">
</div>
</template>
```
2. 在组件的 `setup` 中使用 `ref` 访问元素:
```js
import { ref, onMounted } from 'vue';
export default {
setup() {
const myInput = ref(null);
onMounted(() => {
console.log(myInput.value); // 输出 input 元素对象
});
return {
myInput,
};
},
};
```
在上面的例子中,我们定义了一个 `ref` 对象 `myInput`,然后在 `onMounted` 钩子函数中获取了该元素的对象,并输出到控制台中。
需要注意的是,由于 `ref` 是一个响应式对象,因此在访问元素时需要使用 `myInput.value` 来获取其真实值。
相关问题
vue2获取页面video元素
要获取页面中的 `video` 元素,同样可以使用 `$refs` 属性,方法与获取普通元素相似。
在模板中添加 `ref` 属性:
```html
<video ref="myVideo" src="video.mp4"></video>
```
然后在 Vue 组件中,使用 `$refs` 对象来获取该元素:
```javascript
mounted() {
const videoElement = this.$refs.myVideo;
console.log(videoElement);
}
```
这将输出该 `video` 元素的 DOM 节点,可以使用它来进行操作,比如播放、暂停等等。
vue3如何获取dom元素到页面顶端的距离
在 Vue3 中,获取 DOM 元素到页面顶端的距离可以使用 `ref` 和 `nextTick` 方法。
首先,在模板中给需要获取距离的 DOM 元素绑定一个 `ref`:
```
<template>
<div ref="myElement">Hello World!</div>
</template>
```
然后,在组件中使用 `nextTick` 方法获取该 DOM 元素到页面顶端的距离:
```
import { ref, onMounted, nextTick } from 'vue'
export default {
setup() {
const myElement = ref(null)
onMounted(() => {
nextTick(() => {
const top = myElement.value.getBoundingClientRect().top
console.log(top)
})
})
return {
myElement
}
}
}
```
在 `onMounted` 钩子中,使用 `nextTick` 方法可以确保 DOM 元素已经渲染完成,然后使用 `getBoundingClientRect()` 方法获取该 DOM 元素到页面顶端的距离。
阅读全文
相关推荐
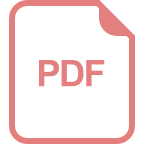
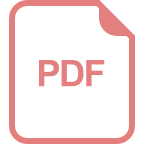
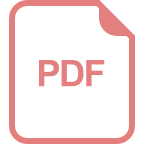
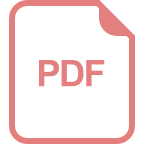











