使用c++ 写出拥有图形化界面的简单聊天室的客户端和服务器代码
时间: 2024-03-05 17:53:53 浏览: 88
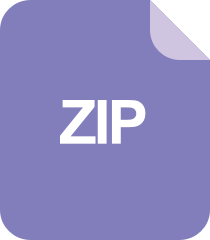
聊天室的客户端和服务器的代码
以下是一个使用C++编写的简单聊天室客户端和服务器端代码,其中使用了Qt框架实现了图形化界面。
客户端代码:
```cpp
#include <QtWidgets>
#include <QtNetwork>
class ChatClient : public QWidget
{
Q_OBJECT
public:
ChatClient(QWidget *parent = 0);
private slots:
void connectToServer();
void sendMessage();
void readMessage();
void displayError(QAbstractSocket::SocketError socketError);
private:
QLineEdit *hostLineEdit;
QLineEdit *portLineEdit;
QLineEdit *messageLineEdit;
QTextEdit *messageBrowser;
QPushButton *connectButton;
QPushButton *sendButton;
QTcpSocket *tcpSocket;
};
ChatClient::ChatClient(QWidget *parent)
: QWidget(parent)
{
// 创建界面元素
hostLineEdit = new QLineEdit("localhost");
portLineEdit = new QLineEdit("12345");
messageLineEdit = new QLineEdit;
messageBrowser = new QTextEdit;
connectButton = new QPushButton("Connect");
sendButton = new QPushButton("Send");
sendButton->setEnabled(false);
// 创建布局
QGridLayout *mainLayout = new QGridLayout;
mainLayout->addWidget(new QLabel("Host:"), 0, 0);
mainLayout->addWidget(hostLineEdit, 0, 1);
mainLayout->addWidget(new QLabel("Port:"), 1, 0);
mainLayout->addWidget(portLineEdit, 1, 1);
mainLayout->addWidget(messageBrowser, 2, 0, 1, 2);
mainLayout->addWidget(messageLineEdit, 3, 0, 1, 2);
mainLayout->addWidget(connectButton, 4, 0);
mainLayout->addWidget(sendButton, 4, 1);
setLayout(mainLayout);
// 创建TCP客户端
tcpSocket = new QTcpSocket(this);
// 连接信号和槽
connect(connectButton, SIGNAL(clicked()), this, SLOT(connectToServer()));
connect(sendButton, SIGNAL(clicked()), this, SLOT(sendMessage()));
connect(tcpSocket, SIGNAL(readyRead()), this, SLOT(readMessage()));
connect(tcpSocket, SIGNAL(error(QAbstractSocket::SocketError)),
this, SLOT(displayError(QAbstractSocket::SocketError)));
}
void ChatClient::connectToServer()
{
// 连接到服务器
tcpSocket->connectToHost(hostLineEdit->text(), portLineEdit->text().toInt());
// 禁用连接按钮
connectButton->setEnabled(false);
}
void ChatClient::sendMessage()
{
// 发送消息
QString message = messageLineEdit->text();
QByteArray data = message.toUtf8();
tcpSocket->write(data);
// 清空输入框
messageLineEdit->clear();
}
void ChatClient::readMessage()
{
// 接收消息
QByteArray data = tcpSocket->readAll();
QString message = QString::fromUtf8(data);
messageBrowser->append(message);
}
void ChatClient::displayError(QAbstractSocket::SocketError socketError)
{
// 显示错误信息
QString message = "Error: " + tcpSocket->errorString();
QMessageBox::warning(this, "Error", message);
// 关闭连接
tcpSocket->abort();
// 启用连接按钮
connectButton->setEnabled(true);
}
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
ChatClient client;
client.show();
return app.exec();
}
```
服务器端代码:
```cpp
#include <QtWidgets>
#include <QtNetwork>
class ChatServer : public QWidget
{
Q_OBJECT
public:
ChatServer(QWidget *parent = 0);
private slots:
void startServer();
void acceptConnection();
void readMessage();
void displayError(QAbstractSocket::SocketError socketError);
private:
QLabel *statusLabel;
QPushButton *startButton;
QTcpServer *tcpServer;
QSet<QTcpSocket *> clients;
};
ChatServer::ChatServer(QWidget *parent)
: QWidget(parent)
{
// 创建界面元素
statusLabel = new QLabel("Status: Not started");
startButton = new QPushButton("Start");
// 创建布局
QVBoxLayout *mainLayout = new QVBoxLayout;
mainLayout->addWidget(statusLabel);
mainLayout->addWidget(startButton);
setLayout(mainLayout);
// 创建TCP服务器
tcpServer = new QTcpServer(this);
// 连接信号和槽
connect(startButton, SIGNAL(clicked()), this, SLOT(startServer()));
connect(tcpServer, SIGNAL(newConnection()), this, SLOT(acceptConnection()));
}
void ChatServer::startServer()
{
// 启动服务器
if (!tcpServer->listen(QHostAddress::Any, 12345)) {
statusLabel->setText("Status: Error");
QMessageBox::warning(this, "Error", tcpServer->errorString());
return;
}
statusLabel->setText("Status: Started");
startButton->setEnabled(false);
}
void ChatServer::acceptConnection()
{
// 接受连接
QTcpSocket *client = tcpServer->nextPendingConnection();
// 添加客户端
clients.insert(client);
// 连接信号和槽
connect(client, SIGNAL(readyRead()), this, SLOT(readMessage()));
connect(client, SIGNAL(disconnected()), client, SLOT(deleteLater()));
connect(client, SIGNAL(disconnected()), this, SLOT(displayError()));
// 发送欢迎消息
QByteArray data = "Welcome to the chat room!\n";
client->write(data);
}
void ChatServer::readMessage()
{
// 接收消息并广播给所有客户端
QTcpSocket *client = qobject_cast<QTcpSocket *>(sender());
QByteArray data = client->readAll();
foreach (QTcpSocket *other, clients) {
if (other != client) {
other->write(data);
}
}
}
void ChatServer::displayError(QAbstractSocket::SocketError socketError)
{
// 显示错误信息
QTcpSocket *client = qobject_cast<QTcpSocket *>(sender());
clients.remove(client);
client->deleteLater();
}
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
ChatServer server;
server.show();
return app.exec();
}
```
需要注意的是,这只是一个简单的聊天室示例,实际应用中需要更加完善的功能和安全性设计。
阅读全文
相关推荐
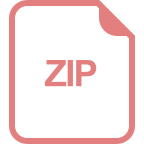
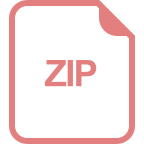
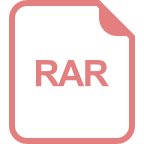
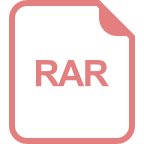
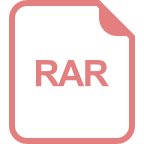
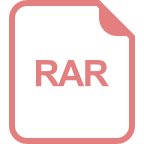
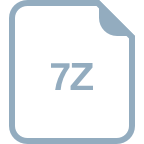
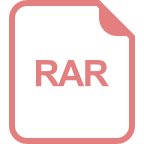
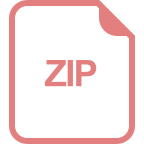
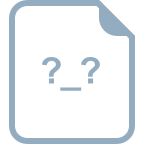
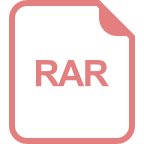
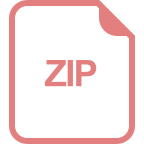
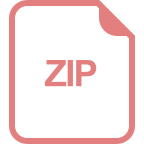
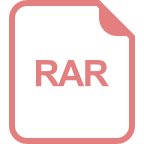
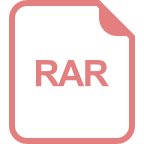
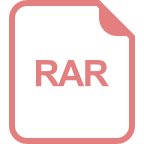