C语言源代码RSA使用平方乘法对输入的数字型消息进行加解密
时间: 2023-07-11 21:06:31 浏览: 81
以下是用C语言实现RSA加解密的代码,使用了平方乘法算法:
```c
#include<stdio.h>
#include<math.h>
unsigned long int gcd(unsigned long int a, unsigned long int b){
if (a == 0)
return b;
return gcd(b % a, a);
}
// 快速幂算法(平方乘法)
unsigned long int modpow(unsigned long int base, unsigned long int exponent, unsigned long int modulus){
unsigned long int result = 1;
while (exponent > 0){
if (exponent%2 == 1){
result = (result * base) % modulus;
}
base = (base * base) % modulus;
exponent = exponent / 2;
}
return result;
}
int main(){
unsigned long int p, q, n, phi, e, d, message, cipher, decrypted;
printf("Enter two prime numbers: ");
scanf("%lu %lu", &p, &q);
n = p*q;
printf("n = %lu\n", n);
phi = (p-1)*(q-1);
printf("phi = %lu\n", phi);
do{
printf("Enter an integer e such that 1 < e < phi and gcd(e,phi) = 1: ");
scanf("%lu", &e);
}while(gcd(e,phi) != 1);
// 计算d
d = 0;
while((d*e)%phi != 1){
d++;
}
printf("d = %lu\n", d);
printf("Enter the message: ");
scanf("%lu", &message);
// 加密
cipher = modpow(message, e, n);
printf("The encrypted message is: %lu\n", cipher);
// 解密
decrypted = modpow(cipher, d, n);
printf("The decrypted message is: %lu\n", decrypted);
return 0;
}
```
在这个代码中,我们使用了 `gcd()` 函数来计算两个数的最大公因数,使用了 `modpow()` 函数来实现快速幂算法(平方乘法)来计算幂模运算。在主函数中,我们先输入两个质数 `p` 和 `q`,计算得到 `n` 和 `phi`。然后输入一个整数 `e`,计算得到 `d`。接着输入需要加密的消息 `message`,使用公钥 `(n,e)` 进行加密,得到密文 `cipher`。最后使用私钥 `(n,d)` 进行解密,得到原始消息 `decrypted`。
阅读全文
相关推荐
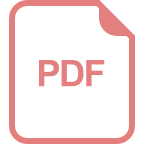
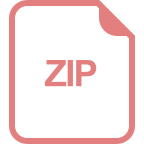
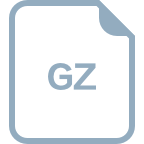
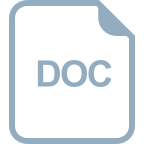
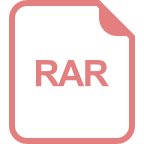
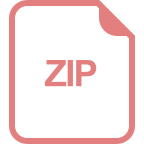
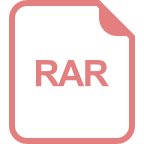
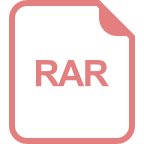
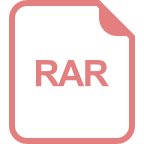
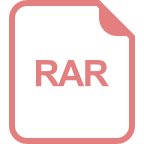
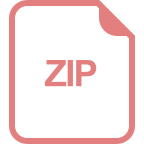
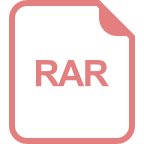
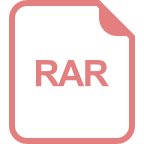
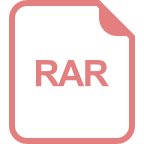
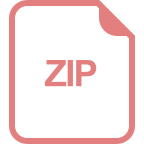