如何在命令行中进入某一个文件路径下
时间: 2024-03-07 16:48:36 浏览: 84
在命令行中进入某一个文件路径下,需要使用 `cd` 命令。`cd` 命令用于更改当前工作目录,让你进入到指定的文件路径下。下面是使用 `cd` 命令的基本语法:
```
cd [目录路径]
```
其中,`[目录路径]` 是要进入的目录路径,可以是相对路径或绝对路径。相对路径是相对于当前工作目录的路径,可以使用 `.` 表示当前目录,使用 `..` 表示上级目录。绝对路径是从根目录开始的完整路径。在 Windows 系统中,路径使用反斜杠 `\` 分隔目录,而在 Linux 和 macOS 系统中,路径使用斜杠 `/` 分隔目录。
例如,要进入 Windows 系统中 D 盘下的 my_folder 目录,可以使用以下命令:
```
cd D:\my_folder
```
要进入 Linux 或 macOS 系统中的 /home/user/my_folder 目录,可以使用以下命令:
```
cd /home/user/my_folder
```
如果要进入当前工作目录的上级目录,可以使用以下命令:
```
cd ..
```
如果要进入当前工作目录的子目录或子文件夹,可以使用以下命令:
```
cd subdirectory
```
注意,在命令行中输入路径时,应该使用正确的路径分隔符,并根据操作系统的不同使用相应的大小写。如果要查看当前工作目录的路径,可以使用 `pwd` 命令(在 Windows 系统中使用 `cd` 命令也可以显示当前工作目录的路径)。
相关问题
C语言写问题描述:编写程序,模拟命令行的常用文件系统管理命令,具体要求如下: ① 根路径为 ROOT。首次进入模拟器时,提示符为“ROOT>”,“>”左侧为当前路径,可在 “>” 后输入下述各命令。 ② 切换到当前路径下的某文件夹:cd 文件夹。如“cd music”,若当前路径下存在 music 文件 夹,则提示符变为“当前路径\music>”,若不存在,则提示。 ③ 切换到当前路径的上级文件夹:cd ..。 ④ 在任意路径下切换回根路径 ROOT:cd \。 ⑤ 列出当前路径下的全部文件夹和文件:dir。 ⑥ 在当前路径下新建文件夹:md 文件夹名称。 ⑦ 在当前路径下新建文件:mf 文件名称。 ⑧ 删除当前路径下的某文件或文件夹(及其下所有文件夹及文件):del 文件或文件夹名称。 注意: ① 以上各命令涉及到的文件夹和文件,仅在内存中用树结构模拟,而非真正的文件系统。 ② 树中结点要包含必要的信息,如:文件或文件夹名称、区分文件或文件夹的标识、文件创建 日期等。 涉及算法及知识:树的创建、遍历、查找、插入、删除。
以下是 C 语言的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#define MAX_PATH_LEN 100 // 文件路径最大长度
#define MAX_FILE_NUM 100 // 文件最大数量
// 文件类型
enum FileType {
File,
Folder
};
// 文件结构体
struct File {
char name[MAX_PATH_LEN]; // 文件名
enum FileType type; // 文件类型
struct File *sub_files[MAX_FILE_NUM]; // 子文件
int sub_file_num; // 子文件数量
time_t create_time; // 创建时间
};
struct File *root; // 根节点
char cur_path[MAX_PATH_LEN] = "ROOT"; // 当前路径
// 创建文件
struct File *create_file(char *name, enum FileType type) {
struct File *file = (struct File *)malloc(sizeof(struct File));
strcpy(file->name, name);
file->type = type;
file->sub_file_num = 0;
file->create_time = time(NULL);
return file;
}
// 删除文件
void delete_file(struct File *file) {
for (int i = 0; i < file->sub_file_num; i++) {
delete_file(file->sub_files[i]);
}
free(file);
}
// 在当前路径下查找文件
struct File *find_file(char *name) {
struct File *cur_file = root;
char *token = strtok(cur_path, "\\");
while (token != NULL) {
for (int i = 0; i < cur_file->sub_file_num; i++) {
if (strcmp(cur_file->sub_files[i]->name, token) == 0) {
cur_file = cur_file->sub_files[i];
break;
}
}
token = strtok(NULL, "\\");
}
for (int i = 0; i < cur_file->sub_file_num; i++) {
if (strcmp(cur_file->sub_files[i]->name, name) == 0) {
return cur_file->sub_files[i];
}
}
return NULL;
}
// 在当前路径下添加文件
void add_file(struct File *file) {
struct File *cur_file = root;
char *token = strtok(cur_path, "\\");
while (token != NULL) {
for (int i = 0; i < cur_file->sub_file_num; i++) {
if (strcmp(cur_file->sub_files[i]->name, token) == 0) {
cur_file = cur_file->sub_files[i];
break;
}
}
token = strtok(NULL, "\\");
}
cur_file->sub_files[cur_file->sub_file_num++] = file;
}
// 列出当前路径下的文件
void list_files() {
struct File *cur_file = root;
char *token = strtok(cur_path, "\\");
while (token != NULL) {
for (int i = 0; i < cur_file->sub_file_num; i++) {
if (strcmp(cur_file->sub_files[i]->name, token) == 0) {
cur_file = cur_file->sub_files[i];
break;
}
}
token = strtok(NULL, "\\");
}
for (int i = 0; i < cur_file->sub_file_num; i++) {
printf("%s %s %s", cur_file->sub_files[i]->type == Folder ? "Folder" : "File",
cur_file->sub_files[i]->name, ctime(&cur_file->sub_files[i]->create_time));
}
}
int main() {
root = create_file("ROOT", Folder);
while (1) {
printf("%s> ", cur_path);
char cmd[10], arg[MAX_PATH_LEN];
scanf("%s%s", cmd, arg);
if (strcmp(cmd, "cd") == 0) {
if (strcmp(arg, "\\") == 0) { // 切换到根目录
strcpy(cur_path, "ROOT");
} else if (strcmp(arg, "..") == 0) { // 切换到上级目录
char *last_slash = strrchr(cur_path, '\\');
if (last_slash != NULL) {
*last_slash = '\0';
}
} else { // 切换到指定目录
struct File *file = find_file(arg);
if (file == NULL || file->type != Folder) {
printf("Folder not found!\n");
} else {
strcat(cur_path, "\\");
strcat(cur_path, arg);
}
}
} else if (strcmp(cmd, "dir") == 0) { // 列出当前目录下的文件
list_files();
} else if (strcmp(cmd, "md") == 0) { // 创建文件夹
struct File *file = create_file(arg, Folder);
add_file(file);
} else if (strcmp(cmd, "mf") == 0) { // 创建文件
struct File *file = create_file(arg, File);
add_file(file);
} else if (strcmp(cmd, "del") == 0) { // 删除文件或文件夹
struct File *file = find_file(arg);
if (file == NULL) {
printf("File not found!\n");
} else {
delete_file(file);
}
} else {
printf("Invalid command!\n");
}
}
return 0;
}
```
以上代码实现了基本的文件系统管理命令,包括切换目录,列出文件,创建文件夹和文件,删除文件和文件夹等操作。在实现过程中,使用了树的结构来模拟文件系统,每个节点包含文件或文件夹的名称、类型、子文件、创建时间等信息。通过遍历树来查找和操作文件。
7z命令行打包文件夹下的某几个文件
要使用7z命令行打包文件夹下的某几个文件,可以按照以下步骤进行操作:
1. 打开命令行(Command Prompt)或终端(Terminal),进入要打包的文件夹所在的目录。
2. 输入以下命令:
```
7z a -tzip archive.zip file1 file2 file3
```
其中,`-tzip`表示要创建zip格式的压缩包,`archive.zip`为要创建的压缩包的名称,`file1 file2 file3`为要打包的文件名。如果要打包整个文件夹,可以使用通配符`*`,例如:
```
7z a -tzip archive.zip *
```
3. 执行命令后,7z将会创建一个名为`archive.zip`的压缩包,其中包含了指定的文件或文件夹。
注意,7z需要事先安装在计算机上,并且需要将7z的安装路径添加到系统环境变量中。另外,上述命令中的参数和选项也可以根据实际需求进行修改。
阅读全文
相关推荐
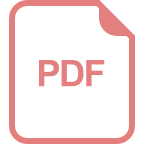
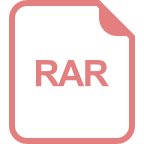
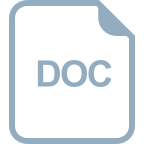
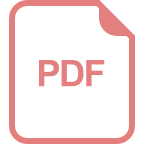
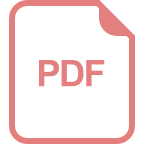
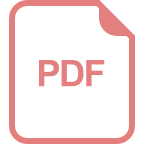
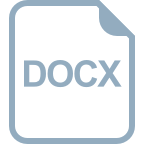
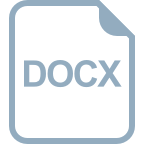
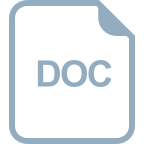
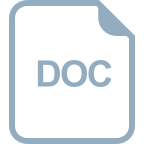
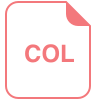
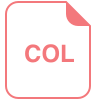
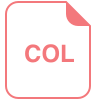
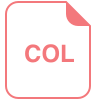
