利用函数补充编写模拟计算器程序,要求含有加法、减法、乘法、除法、幂运算、取余和取整等运算。每个运算为一个函数,且程序循环获取用户的运算请求,直到用户确认退出,程序结束。 功能(主菜单)如下: 1:加法 2:减法 3:乘法 4:除法 5:幂运算 6:取余 7:开平方 q:退出
时间: 2023-06-13 20:04:58 浏览: 125
可以使用Python来实现这个计算器程序,以下是代码实现:
```python
import math
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
def divide(x, y):
if y == 0:
return "Error: divide by zero"
else:
return x / y
def power(x, y):
return x ** y
def remainder(x, y):
if y == 0:
return "Error: divide by zero"
else:
return x % y
def square_root(x):
if x < 0:
return "Error: cannot take square root of negative number"
else:
return math.sqrt(x)
def get_user_input():
operation = input("Enter operation (+, -, *, /, **, %, sqrt, q to quit): ")
if operation == "q":
return (operation, None, None)
elif operation == "sqrt":
x = float(input("Enter a number: "))
return (operation, x, None)
else:
x = float(input("Enter first number: "))
y = float(input("Enter second number: "))
return (operation, x, y)
def perform_operation(operation, x, y):
if operation == "+":
result = add(x, y)
elif operation == "-":
result = subtract(x, y)
elif operation == "*":
result = multiply(x, y)
elif operation == "/":
result = divide(x, y)
elif operation == "**":
result = power(x, y)
elif operation == "%":
result = remainder(x, y)
elif operation == "sqrt":
result = square_root(x)
return result
while True:
operation, x, y = get_user_input()
if operation == "q":
break
else:
result = perform_operation(operation, x, y)
print("Result: ", result)
```
运行程序后,会进入一个循环,每次获取用户输入的运算请求,然后调用对应的函数进行计算,最后输出结果。用户可以输入“q”来退出程序。
相关推荐
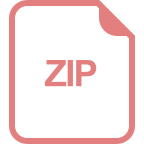
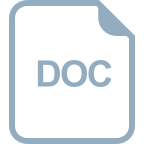
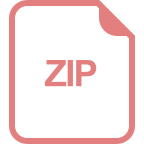












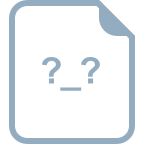
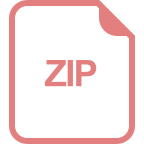
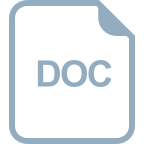