javafx如何利用多个线程加载目录树中的图片文件
时间: 2024-05-10 14:19:33 浏览: 4
JavaFX应用程序可以使用多个线程来加载目录树中的图片文件。以下是一些步骤:
1. 创建一个线程池,该线程池将负责加载图像文件。您可以使用JavaFX的内置线程池类- `javafx.concurrent.WorkerThread`。
2. 在UI线程中,使用`Platform.runLater()`方法将任务提交到JavaFX应用程序的事件队列中,以便在UI线程中处理UI更新。
3. 使用`Files.walk()`方法遍历目录树中的所有图像文件,并将它们添加到待加载的文件列表中。
4. 在线程池中,使用`ImageIO.read()`方法读取每个文件的内容,并将其转换为JavaFX的`Image`对象。
5. 在加载完成后,使用`Platform.runLater()`方法将加载的图像添加到UI中。
以下是一个示例代码片段,它使用多个线程加载目录树中的所有图像,并将它们添加到JavaFX应用程序中:
```java
ExecutorService executor = Executors.newFixedThreadPool(4);
// Traverse the directory tree and create a list of image files to load
List<Path> imagePaths = new ArrayList<>();
Files.walk(Paths.get("path/to/directory"))
.filter(path -> Files.isRegularFile(path) && isImageFile(path))
.forEach(imagePaths::add);
// Load the images in a background thread
for (Path imagePath : imagePaths) {
executor.submit(() -> {
try {
// Load the image
BufferedImage bufferedImage = ImageIO.read(imagePath.toFile());
Image image = SwingFXUtils.toFXImage(bufferedImage, null);
// Update the UI thread with the loaded image
Platform.runLater(() -> {
// Add the image to the UI
// ...
});
} catch (IOException e) {
e.printStackTrace();
}
});
}
// Helper method to check if a file is an image file
private boolean isImageFile(Path path) {
String extension = StringUtils.getFilenameExtension(path.toString());
return extension != null && ImageIO.getImageReadersBySuffix(extension).hasNext();
}
```
在这个例子中,我们使用了一个固定大小的线程池来加载图像文件。我们使用`Files.walk()`方法遍历目录树中的所有图像文件,并将它们添加到待加载的文件列表中。然后,我们在执行线程池中为每个图像文件提交一个任务。在任务中,我们使用`ImageIO.read()`方法读取图像文件的内容,并将其转换为JavaFX的`Image`对象。最后,我们使用`Platform.runLater()`方法将加载的图像添加到UI中。
相关推荐
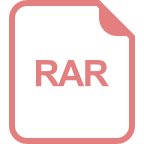
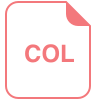
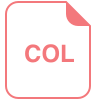
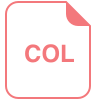











