ds2740的c51程序
时间: 2023-09-28 14:05:52 浏览: 58
以下是一个简单的C51程序,用于读取DS2740芯片的电流和温度数据。这个程序假设DS2740芯片的I2C地址是0x2C,并且使用I2C总线。请注意,您需要根据您的硬件配置和应用程序的需求修改此程序。
```c
#include <reg52.h>
#include <intrins.h>
#define DS2740_ADDRESS 0x2C
void i2c_start();
void i2c_stop();
void i2c_write(unsigned char dat);
unsigned char i2c_read(unsigned char ack);
void delay(unsigned int ms);
void main() {
unsigned char msb, lsb;
int current;
unsigned char temperature;
while(1) {
i2c_start();
i2c_write(DS2740_ADDRESS << 1);
i2c_write(0x00); // Select Current Register
i2c_start();
i2c_write((DS2740_ADDRESS << 1) | 0x01);
msb = i2c_read(1);
lsb = i2c_read(0);
i2c_stop();
current = (msb << 8) | lsb;
current = current / 10; // Convert to milliamps
i2c_start();
i2c_write(DS2740_ADDRESS << 1);
i2c_write(0x01); // Select Temperature Register
i2c_start();
i2c_write((DS2740_ADDRESS << 1) | 0x01);
temperature = i2c_read(0);
i2c_stop();
printf("Current: %d mA, Temperature: %d C\n", current, temperature);
delay(1000);
}
}
void i2c_start() {
SDA = 1;
SCL = 1;
_nop_();
SDA = 0;
_nop_();
SCL = 0;
}
void i2c_stop() {
SDA = 0;
SCL = 1;
_nop_();
SDA = 1;
_nop_();
SCL = 0;
}
void i2c_write(unsigned char dat) {
unsigned char i;
for(i = 0; i < 8; i++) {
SDA = (dat & 0x80) >> 7;
dat <<= 1;
_nop_();
SCL = 1;
_nop_();
SCL = 0;
}
SDA = 1;
_nop_();
SCL = 1;
_nop_();
SCL = 0;
}
unsigned char i2c_read(unsigned char ack) {
unsigned char i, dat = 0;
SDA = 1;
for(i = 0; i < 8; i++) {
dat <<= 1;
SCL = 1;
_nop_();
dat |= SDA;
_nop_();
SCL = 0;
}
SDA = ack;
_nop_();
SCL = 1;
_nop_();
SCL = 0;
return dat;
}
void delay(unsigned int ms) {
unsigned int i, j;
for(i = 0; i < ms; i++) {
for(j = 0; j < 1000; j++);
}
}
```
该程序使用C51单片机的GPIO口模拟I2C总线与DS2740芯片通信,并读取电流和温度数据。在程序中,i2c_start()、i2c_stop()、i2c_write()和i2c_read()函数实现了I2C总线的基本操作。该程序使用printf()函数将电流和温度数据输出到串口。请注意,您需要在编译和烧写程序之前配置您的开发环境。
相关推荐















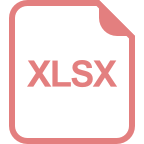