使用c#语言实现功能:1、读取properties文件;2、修改properties文件指定的属性值
时间: 2024-05-04 10:20:17 浏览: 14
你可以使用C#中的`System.IO`和`System.Collections.Generic`命名空间来实现读取和修改properties文件中的属性值。
1. 读取properties文件
可以使用`System.IO.StreamReader`类来读取properties文件中的内容,然后使用`System.Collections.Generic.Dictionary`类将内容转换为键值对。
```csharp
using System.IO;
using System.Collections.Generic;
public Dictionary<string, string> ReadProperties(string filePath)
{
Dictionary<string, string> properties = new Dictionary<string, string>();
using (StreamReader reader = new StreamReader(filePath))
{
while (!reader.EndOfStream)
{
string line = reader.ReadLine().Trim();
if (!string.IsNullOrEmpty(line) && !line.StartsWith("#"))
{
int index = line.IndexOf('=');
if (index > 0 && index < line.Length - 1)
{
string key = line.Substring(0, index).Trim();
string value = line.Substring(index + 1).Trim();
if (!properties.ContainsKey(key))
{
properties.Add(key, value);
}
}
}
}
}
return properties;
}
```
上述代码将会返回一个包含properties文件中所有属性的键值对字典。
2. 修改properties文件指定的属性值
可以使用`System.IO.StreamWriter`类来写入修改后的内容到properties文件中。
```csharp
using System.IO;
public void ModifyProperty(string filePath, string key, string value)
{
string tempFilePath = filePath + ".tmp";
using (StreamReader reader = new StreamReader(filePath))
{
using (StreamWriter writer = new StreamWriter(tempFilePath))
{
while (!reader.EndOfStream)
{
string line = reader.ReadLine().Trim();
if (!string.IsNullOrEmpty(line) && !line.StartsWith("#"))
{
int index = line.IndexOf('=');
if (index > 0 && index < line.Length - 1)
{
string currentKey = line.Substring(0, index).Trim();
if (currentKey == key)
{
line = string.Format("{0}={1}", key, value);
}
}
writer.WriteLine(line);
}
}
}
}
File.Delete(filePath);
File.Move(tempFilePath, filePath);
}
```
上述代码将会修改properties文件中指定键对应的属性值。注意,这里使用了一个临时文件来保存修改后的内容,如果修改成功,需要删除原来的文件并重命名临时文件为原来的文件名。
相关推荐
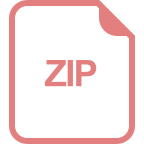









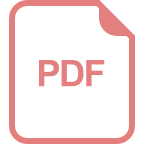