用python的for循环来删除重复字符
时间: 2023-03-29 09:00:57 浏览: 82
可以使用以下代码来删除字符串中的重复字符:
```
str = "hello world"
new_str = ""
for char in str:
if char not in new_str:
new_str += char
print(new_str)
```
输出结果为: "helo wrd"
相关问题
python删除重复字符
你可以使用以下代码来删除字符串中的重复字符:
```python
def remove_duplicates(string):
# 将字符串转换为列表
string_list = list(string)
# 创建一个空集合
seen = set()
# 用一个循环遍历列表中的每个字符
# 如果这个字符不在集合中,就将其加入集合并添加到新列表中
# 否则,忽略它
new_list = []
for char in string_list:
if char not in seen:
seen.add(char)
new_list.append(char)
# 将新列表转换为字符串并返回
return ''.join(new_list)
```
你可以将要删除重复字符的字符串作为参数传递给上述函数。
Python编写程序,输入一个字符串“Hello This is Python.”删除其中的重复空格,使用字符串切片和循环
在Python中,你可以通过遍历字符串并利用字符串切片的方式来删除重复的空格。这里是一个简单的例子:
```python
def remove_duplicates(input_string):
# 使用split()函数将字符串分割成单词列表,同时移除空格
words = input_string.split()
# 初始化一个新的变量,用于存储处理后的无重复空格的字符串
result = []
# 遍历单词列表
for word in words:
# 检查当前单词前是否还有空格,如果有则添加一个空格到结果中
if result and not result[-1] == ' ':
result.append(' ')
# 添加单词到结果
result.append(word)
# 再次使用join()函数合并单词,此时已自动去除重复空格
cleaned_string = ''.join(result)
return cleaned_string
# 测试上述函数
input_string = "Hello This is Python."
output_string = remove_duplicates(input_string)
print("原字符串:", input_string)
print("处理后的字符串:", output_string)
阅读全文
相关推荐
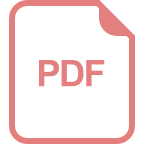
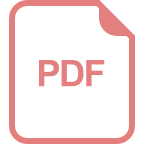
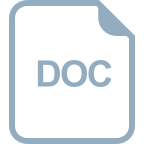

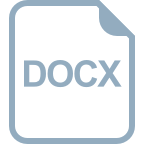
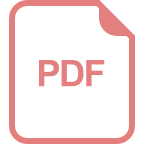
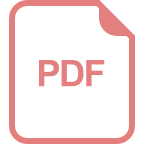
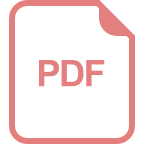
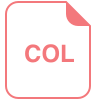
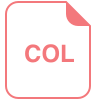
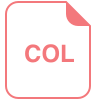
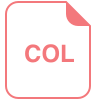



