使用putchar输出一段英文
时间: 2023-05-18 15:04:24 浏览: 61
Sure, here's an example of using putchar to output a string of English text:
```
#include <stdio.h>
int main() {
char str[] = "Hello, world!";
int i = 0;
while (str[i] != '\0') {
putchar(str[i]);
i++;
}
return 0;
}
```
This program declares a string variable `str` containing the text "Hello, world!", and then uses a loop to iterate over each character in the string and output it using putchar. The loop continues until it reaches the null terminator character '\0', which marks the end of the string.
相关问题
c语言中使用putchar输出hello world
你好,以下是回答:
可以使用以下代码在 C 语言中使用 putchar 输出 "hello world":
#include <stdio.h>
int main() {
putchar('h');
putchar('e');
putchar('l');
putchar('l');
putchar('o');
putchar(' ');
putchar('w');
putchar('o');
putchar('r');
putchar('l');
putchar('d');
return ;
}
输出结果为:hello world
c语言putchar一次输出一个字符
### 回答1:
是的,C语言中的putchar函数可以一次输出一个字符。它的函数原型为:
```c
int putchar(int c);
```
其中,参数c是要输出的字符。该函数会将c输出到标准输出流(通常是屏幕),并返回输出的字符的ASCII码值。例如,要输出字符'A',可以使用如下代码:
```c
putchar('A');
```
这将会在屏幕上输出字母A。
### 回答2:
C语言中的putchar函数是用来将一个字符输出到标准输出流(通常是显示器)上的函数。它一次只能输出一个字符。
使用putchar函数非常简单,只需要在函数中传入一个字符作为参数即可。例如,如果我们想要输出字符'a',则可以使用以下代码:
```
putchar('a');
```
这样,字符'a'就会被输出到屏幕上。
putchar函数的返回值类型是int型,它会返回输出的字符。因此,我们还可以通过将putchar函数的返回值赋值给一个变量来获取输出的字符。例如:
```
int ch = putchar('a');
```
这样,变量ch将会被赋值为字符'a'的ASCII码,而字符'a'也会被输出到屏幕上。
需要注意的是,由于putchar函数只输出一个字符,如果想要输出多个字符,我们需要多次调用putchar函数。例如,如果想要输出字符串"Hello",我们可以使用以下代码:
```
putchar('H');
putchar('e');
putchar('l');
putchar('l');
putchar('o');
```
这样,字符串"Hello"就会被逐个字符地输出到屏幕上。
总结起来,C语言的putchar函数可以一次输出一个字符到标准输出流,通过多次调用putchar函数可以逐个输出多个字符。
### 回答3:
C语言的putchar函数用于将一个字符输出到标准输出设备。它一次只能输出一个字符。
putchar函数的使用非常简单,只需要将要输出的字符作为参数传递给它即可。例如,要输出字符'A',可以使用如下代码:
```c
putchar('A');
```
该代码将字符'A'输出到标准输出设备,即控制台上。
除了直接使用字符常量作为参数,也可以使用变量来传递字符给putchar函数。例如,要输出变量ch中存储的字符,可以使用如下代码:
```c
char ch = 'B';
putchar(ch);
```
该代码将变量ch中的字符'B'输出到标准输出设备。
需要注意的是,putchar函数只能输出一个字符,并不接受格式化字符串。
总结:C语言的putchar函数用于一次输出一个字符到标准输出设备,可以使用直接的字符常量或者变量作为参数。
相关推荐
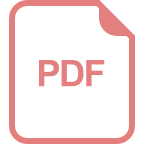
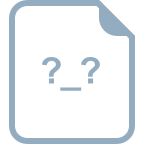
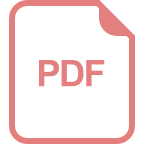












