写一个多线程应用程序,要求能在用户级实现线程的调度,如启动、挂起、恢复、停止,通过pthread实现并解释。
时间: 2024-04-30 13:23:43 浏览: 101
以下是一个使用pthread实现的多线程应用程序,该程序能够在用户级实现线程的调度,包括启动、挂起、恢复、停止。该程序使用了互斥锁和条件变量来实现线程的同步和控制。
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <unistd.h>
// 线程结构体
typedef struct {
pthread_t tid; // 线程ID
int state; // 线程状态,0:未启动,1:运行中,2:挂起,3:停止
} thread_t;
// 线程函数
void *thread_func(void *arg)
{
thread_t *t = (thread_t *)arg;
while (1) {
// 等待唤醒信号
pthread_mutex_lock(&mutex);
while (t->state == 2) {
pthread_cond_wait(&cond, &mutex);
}
pthread_mutex_unlock(&mutex);
// 执行线程函数
printf("Thread %lu is running...\n", t->tid);
sleep(1);
// 判断线程状态
if (t->state == 3) {
printf("Thread %lu is stopped.\n", t->tid);
break;
}
}
return NULL;
}
// 线程控制函数
void thread_control(thread_t *t, int cmd)
{
switch (cmd) {
case 0: // 启动线程
if (t->state == 0) {
pthread_create(&t->tid, NULL, thread_func, t);
t->state = 1;
printf("Thread %lu is started.\n", t->tid);
}
break;
case 1: // 挂起线程
if (t->state == 1) {
pthread_mutex_lock(&mutex);
t->state = 2;
printf("Thread %lu is suspended.\n", t->tid);
pthread_mutex_unlock(&mutex);
}
break;
case 2: // 恢复线程
if (t->state == 2) {
pthread_mutex_lock(&mutex);
t->state = 1;
printf("Thread %lu is resumed.\n", t->tid);
pthread_cond_signal(&cond);
pthread_mutex_unlock(&mutex);
}
break;
case 3: // 停止线程
if (t->state == 1 || t->state == 2) {
pthread_mutex_lock(&mutex);
t->state = 3;
printf("Thread %lu is stopping...\n", t->tid);
pthread_cond_signal(&cond);
pthread_mutex_unlock(&mutex);
pthread_join(t->tid, NULL);
}
break;
default:
break;
}
}
// 全局变量
thread_t t1 = {0, 0};
thread_t t2 = {0, 0};
pthread_mutex_t mutex;
pthread_cond_t cond;
int main()
{
// 初始化互斥锁和条件变量
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&cond, NULL);
// 启动线程1和线程2
thread_control(&t1, 0);
thread_control(&t2, 0);
// 挂起线程1
thread_control(&t1, 1);
// 恢复线程1
thread_control(&t1, 2);
// 停止线程1和线程2
thread_control(&t1, 3);
thread_control(&t2, 3);
// 销毁互斥锁和条件变量
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&cond);
return 0;
}
```
该程序定义了一个线程结构体,包括线程ID和线程状态。线程函数中使用了互斥锁和条件变量来实现线程的同步和控制,当线程状态为2时,线程进入等待状态,等待唤醒信号;当线程状态为3时,线程退出循环并停止执行。
该程序还定义了一个线程控制函数,可以用来启动、挂起、恢复和停止线程。当需要启动线程时,调用线程控制函数并传入0;当需要挂起线程时,调用线程控制函数并传入1;当需要恢复线程时,调用线程控制函数并传入2;当需要停止线程时,调用线程控制函数并传入3。
在main函数中,先初始化互斥锁和条件变量,然后启动线程1和线程2,接着挂起线程1并恢复线程1,最后停止线程1和线程2,并销毁互斥锁和条件变量。
阅读全文
相关推荐




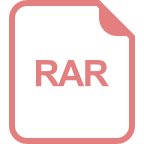













