Python如何从字符串中提取子串?
时间: 2024-09-09 15:05:31 浏览: 22
在Python中,可以使用内置函数和切片操作来从字符串中提取子串。这里有几种常见的方法:
1. **使用索引**:通过指定开始和结束位置(包括开始但不包括结束),你可以获取子串。例如:
```python
string = "Hello, World!"
substring = string[7:12] # 提取"World"
```
2. **切片**:使用`[:]`操作符加上起始和结束范围(省略结束表示到字符串末尾)。负索引用于倒数计数:
```python
substring = string[:5] # 提取前五个字符,即"Hello"
substring = string[-6:] # 提取最后六个字符,即"World!"
```
3. **`str.split()`** 或 `str.partition()` 等方法:如果需要按特定分隔符提取子串,可以使用这些函数。如:
```python
words = string.split(",") # 分割逗号,返回一个列表
```
4. **正则表达式**(`re`模块):对于复杂的模式匹配,可以使用`re.search()`、`re.findall()`等方法。
相关问题
用正则表达式从一串字符串中提取子串并存入字典中
好的,我可以为你解答这个问题。
首先,正则表达式是一种用于匹配文本的工具,可以用来从一串字符串中提取子串。具体来说,可以使用 Python 中的 `re` 模块来进行正则表达式的操作。
下面是一个示例代码,可以从一段文本中提取出所有的邮箱地址,并将其存储到一个字典中,以邮箱地址作为字典的 key,出现次数作为 value:
```python
import re
text = "john@example.com, mary@gmail.com, john@example.com"
email_dict = {}
pattern = r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b'
matches = re.findall(pattern, text)
for match in matches:
if match in email_dict:
email_dict[match] += 1
else:
email_dict[match] = 1
print(email_dict)
```
在上面的代码中,我们首先定义了一个字符串 `text`,其中包含了若干个邮箱地址。接着,我们定义了一个空字典 `email_dict`,用于存储提取出来的邮箱地址及其出现次数。然后,我们使用正则表达式 `r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b'` 来匹配出所有的邮箱地址,使用 `re.findall()` 函数将匹配结果存储到 `matches` 变量中。最后,我们遍历 `matches` 中的每个匹配结果,将其存储到 `email_dict` 中,并计算每个邮箱地址出现的次数。
希望这个示例代码可以帮助你更好地理解如何使用正则表达式从一串字符串中提取子串,并将其存储到字典中。
从字符串中提取最长的数字子串
可以使用正则表达式来实现从字符串中提取最长的数字子串。具体做法是,使用正则表达式匹配字符串中的所有数字子串,然后取出其中最长的一个即可。
以下是 Python 代码示例:
```python
import re
def extract_longest_number_substring(s):
pattern = r'\d+'
matches = re.findall(pattern, s)
if not matches:
return ''
longest_match = max(matches, key=len)
return longest_match
# 示例
s = 'ab123cd5678ef9'
longest_number_substring = extract_longest_number_substring(s)
print(longest_number_substring) # 输出:5678
```
在上面的代码中,我们使用 `re.findall()` 函数来查找字符串 `s` 中所有的数字子串,然后使用 `max()` 函数取出其中最长的一个。如果 `findall()` 函数没有找到任何数字子串,那么说明原字符串中没有数字,此时函数返回空字符串 `''`。
相关推荐
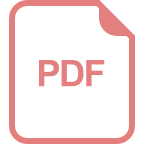
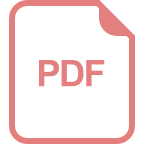
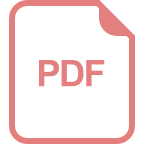












