named pipe是什么
时间: 2024-02-26 09:41:29 浏览: 27
named pipe,也叫做FIFO(First In, First Out),是一种进程间通信(IPC)机制,用于在两个进程之间传输数据。与匿名管道不同,命名管道可以被多个进程同时使用,并且可以在文件系统中持久化存储。命名管道通常通过mkfifo()函数创建,并以文件名的形式存在于文件系统中。在Linux系统中,命名管道的文件类型为p。可以使用标准I/O函数(如read()、write()等)来读写命名管道。
相关问题
windows进程通信机制采用有名管道;程序分两个部分,即Named pipe server和Named pipe client。设计代码并运行
### 回答1:
以下是一个简单的 Named Pipe Server 和 Named Pipe Client 的示例代码,使用 Windows API 实现了进程间通信:
Named Pipe Server:
```c++
#include <windows.h>
#include <stdio.h>
int main()
{
HANDLE hPipe;
char buffer[1024];
DWORD dwRead;
// 创建命名管道
hPipe = CreateNamedPipe(TEXT("\\\\.\\pipe\\MyPipe"), PIPE_ACCESS_DUPLEX, PIPE_TYPE_BYTE | PIPE_READMODE_BYTE | PIPE_WAIT, 1, 1024, 1024, NMPWAIT_USE_DEFAULT_WAIT, NULL);
// 等待客户端连接
ConnectNamedPipe(hPipe, NULL);
// 从管道中读取数据
ReadFile(hPipe, buffer, sizeof(buffer), &dwRead, NULL);
buffer[dwRead] = '\0';
// 输出读取的数据
printf("Received message: %s\n", buffer);
// 关闭管道句柄
CloseHandle(hPipe);
return 0;
}
```
Named Pipe Client:
```c++
#include <windows.h>
#include <stdio.h>
int main()
{
HANDLE hPipe;
char buffer[1024] = "Hello, Named Pipe!";
DWORD dwWritten;
// 连接到命名管道
hPipe = CreateFile(TEXT("\\\\.\\pipe\\MyPipe"), GENERIC_READ | GENERIC_WRITE, 0, NULL, OPEN_EXISTING, 0, NULL);
// 向管道中写入数据
WriteFile(hPipe, buffer, strlen(buffer), &dwWritten, NULL);
// 关闭管道句柄
CloseHandle(hPipe);
return 0;
}
```
使用 Visual Studio 将上述代码编译成可执行文件,然后先运行 Named Pipe Server,再运行 Named Pipe Client,可以看到 Named Pipe Server 接收到了来自 Named Pipe Client 发送的消息。
### 回答2:
Windows进程通信机制采用有名管道是一种非常常见的方法。有名管道允许不同进程之间进行双向通信,其中一个进程作为管道的服务器,另一个进程作为管道的客户端。
下面是一个简单的示例,展示了如何使用有名管道来实现进程通信:
`Named pipe server`
```c++
#include<iostream>
#include<Windows.h>
int main()
{
HANDLE hPipe;
LPTSTR lpPipeName = TEXT("\\\\.\\pipe\\MyPipe");
// 创建有名管道
hPipe = CreateNamedPipe(
lpPipeName,
PIPE_ACCESS_DUPLEX,
PIPE_TYPE_BYTE | PIPE_READMODE_BYTE | PIPE_WAIT,
PIPE_UNLIMITED_INSTANCES,
512,
512,
0,
NULL);
if (hPipe == INVALID_HANDLE_VALUE)
{
std::cout << "无法创建管道" << std::endl;
return 1;
}
// 与客户端建立连接
std::cout << "等待客户端连接..." << std::endl;
ConnectNamedPipe(hPipe, NULL);
std::cout << "客户端已连接" << std::endl;
// 向客户端发送消息
char szMessage[] = "Hello, client!";
DWORD dwWritten;
WriteFile(hPipe, szMessage, sizeof(szMessage), &dwWritten, NULL);
// 关闭管道
DisconnectNamedPipe(hPipe);
CloseHandle(hPipe);
return 0;
}
```
`Named pipe client`
```c++
#include<iostream>
#include<Windows.h>
int main()
{
HANDLE hPipe;
LPTSTR lpPipeName = TEXT("\\\\.\\pipe\\MyPipe");
char szMessage[512];
DWORD dwRead;
// 连接到服务器管道
hPipe = CreateFile(
lpPipeName,
GENERIC_READ | GENERIC_WRITE,
0,
NULL,
OPEN_EXISTING,
0,
NULL);
if (hPipe == INVALID_HANDLE_VALUE)
{
std::cout << "无法连接到管道服务器" << std::endl;
return 1;
}
// 从服务器接收消息
ReadFile(hPipe, szMessage, sizeof(szMessage), &dwRead, NULL);
std::cout << "从服务器接收到消息:" << szMessage << std::endl;
// 关闭管道
CloseHandle(hPipe);
return 0;
}
```
上面的代码演示了一个非常简单的例子,其中 Named pipe server 创建了一个有名管道,并等待客户端的连接。一旦客户端连接成功,它向客户端发送一条消息。 Named pipe client 连接到服务器,接收来自服务器的消息。
运行这两个程序将会实现进程间的通信。首先运行 Named pipe server,然后再运行 Named pipe client。你将看到服务器程序等待客户端连接,并发送消息给客户端,客户端程序接收并显示服务器发送的消息。
这只是一个简单的示例,你可以根据需求进行更复杂的通信操作。
### 回答3:
Windows进程通信机制采用有名管道是一种进程之间进行通信的方式,可以在同一台计算机或网络中的不同计算机上实现进程间通信。
有名管道(Named pipe)是一种特殊的文件,用于进程之间的双向通信。它可以实现在不同的进程中,通过读写管道文件来进行数据的交换。
以下是一个简单的示例代码,分为Named pipe server和Named pipe client两个部分:
Named pipe server代码:
```
#include <windows.h>
#include <stdio.h>
#define PIPE_NAME L"\\\\.\\pipe\\MyPipe"
int main()
{
HANDLE hPipe;
char buffer[1024];
DWORD dwRead;
// 创建有名管道
hPipe = CreateNamedPipe(PIPE_NAME, PIPE_ACCESS_DUPLEX, PIPE_TYPE_MESSAGE | PIPE_READMODE_MESSAGE | PIPE_WAIT, PIPE_UNLIMITED_INSTANCES, 1024, 1024, NMPWAIT_USE_DEFAULT_WAIT, NULL);
if (hPipe == INVALID_HANDLE_VALUE)
{
printf("创建管道失败\n");
return 1;
}
// 等待客户端连接
ConnectNamedPipe(hPipe, NULL);
// 从管道中读取数据
ReadFile(hPipe, buffer, sizeof(buffer), &dwRead, NULL);
buffer[dwRead / sizeof(TCHAR)] = '\0';
printf("接收到的数据:%s\n", buffer);
// 关闭管道
DisconnectNamedPipe(hPipe);
CloseHandle(hPipe);
return 0;
}
```
Named pipe client代码:
```
#include <windows.h>
#include <stdio.h>
#define PIPE_NAME L"\\\\.\\pipe\\MyPipe"
int main()
{
HANDLE hPipe;
char buffer[1024];
DWORD dwWritten;
// 连接到已有的有名管道
hPipe = CreateFile(PIPE_NAME, GENERIC_READ | GENERIC_WRITE, 0, NULL, OPEN_EXISTING, 0, NULL);
if (hPipe == INVALID_HANDLE_VALUE)
{
printf("连接管道失败\n");
return 1;
}
// 向管道写入数据
sprintf(buffer, "Hello Pipe");
WriteFile(hPipe, buffer, strlen(buffer), &dwWritten, NULL);
// 关闭管道
CloseHandle(hPipe);
return 0;
}
```
运行这两个程序,Named pipe server会等待Named pipe client连接,并接收从client发送的数据,并将其打印出来。client会向server发送数据"Hello Pipe"。
c#pipe
C#中的管道(pipe)是一种进程间通信(IPC)的机制,它允许一个进程向另一个进程发送数据。在C#中,可以使用命名管道(named pipes)或匿名管道(anonymous pipes)来实现进程间通信。
命名管道允许在不同的进程之间进行通信,而匿名管道只能在父进程和子进程之间进行通信。命名管道是通过一个唯一的名称来标识的,而匿名管道则是由操作系统自动生成的。
C#中可以使用System.IO.Pipes命名空间来创建和使用管道。例如,以下代码创建一个命名管道:
```csharp
using System.IO.Pipes;
NamedPipeServerStream pipeServer = new NamedPipeServerStream("testpipe", PipeDirection.InOut);
```
这个代码创建了一个名为“testpipe”的命名管道,并设置了管道的方向为双向。接下来,可以使用pipeServer对象来读取和写入数据。
相关推荐
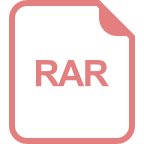
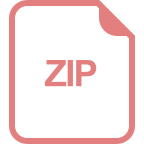












