uniapp 数组循环 下拉菜单筛选 hover 显示 点击选中 下拉菜单 恢复
时间: 2024-02-12 17:06:31 浏览: 31
你的问题比较复杂,需要分开回答。
1. uniapp 数组循环
在uniapp中,你可以使用 v-for 指令来循环一个数组,例如:
```
<template>
<ul>
<li v-for="(item, index) in items" :key="index">{{ item }}</li>
</ul>
</template>
<script>
export default {
data() {
return {
items: ['apple', 'banana', 'orange']
}
}
}
</script>
```
2. 下拉菜单筛选
你可以使用 uni-popup 组件来实现下拉菜单,然后在该组件中添加筛选条件,例如:
```
<template>
<div>
<button @click="showPopup">筛选</button>
<uni-popup v-model="show" :style="{height: '300rpx'}">
<ul>
<li v-for="(item, index) in items" :key="index" v-show="filterItem(item)">{{ item }}</li>
</ul>
</uni-popup>
</div>
</template>
<script>
export default {
data() {
return {
items: ['apple', 'banana', 'orange'],
show: false,
filterValue: ''
}
},
methods: {
showPopup() {
this.show = true
},
filterItem(item) {
return item.includes(this.filterValue)
}
}
}
</script>
```
在上面的代码中,我们使用 uni-popup 组件来实现下拉菜单,然后在下拉菜单中循环 items 数组,使用 v-show 指令来控制每个 li 元素是否显示,filterItem 方法用于筛选符合条件的项,filterValue 是筛选条件的值。
3. hover 显示
你可以使用 CSS 的 :hover 伪类来实现 hover 显示,例如:
```
<template>
<div>
<ul>
<li v-for="(item, index) in items" :key="index">{{ item }}</li>
</ul>
</div>
</template>
<style>
li:hover {
background-color: #eee;
}
</style>
```
在上面的代码中,我们使用 CSS 的 :hover 伪类来实现鼠标悬停时 li 元素的背景色变化。
4. 点击选中
你可以使用 v-bind:class 指令来控制元素的 class,然后在点击时给选中的元素添加特定的 class,例如:
```
<template>
<div>
<ul>
<li v-for="(item, index) in items" :key="index" :class="{active: index === selectedIndex}" @click="selectItem(index)">{{ item }}</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
items: ['apple', 'banana', 'orange'],
selectedIndex: -1
}
},
methods: {
selectItem(index) {
this.selectedIndex = index
}
}
}
</script>
<style>
.active {
background-color: #eee;
}
</style>
```
在上面的代码中,我们使用 v-bind:class 指令来控制 li 元素的 class,根据 selectedIndex 的值来判断当前 li 是否被选中,selectItem 方法用于选中 li 元素。
5. 下拉菜单恢复
你可以在组件的生命周期钩子函数中重置相关的数据,例如:
```
<template>
<div>
<button @click="showPopup">筛选</button>
<uni-popup v-model="show" :style="{height: '300rpx'}">
<ul>
<li v-for="(item, index) in items" :key="index" v-show="filterItem(item)" :class="{active: index === selectedIndex}" @click="selectItem(index)">{{ item }}</li>
</ul>
</uni-popup>
</div>
</template>
<script>
export default {
data() {
return {
items: ['apple', 'banana', 'orange'],
show: false,
filterValue: '',
selectedIndex: -1
}
},
methods: {
showPopup() {
this.show = true
},
filterItem(item) {
return item.includes(this.filterValue)
},
selectItem(index) {
this.selectedIndex = index
this.show = false
}
},
beforeDestroy() {
this.selectedIndex = -1
}
}
</script>
<style>
.active {
background-color: #eee;
}
</style>
```
在上面的代码中,我们在组件的 beforeDestroy 生命周期钩子函数中重置 selectedIndex 的值,确保每次重新打开下拉菜单时 selectedIndex 的值都是 -1。
相关推荐
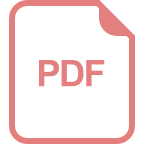
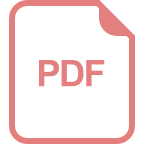














