cursor.fetchall()的结果里的数据怎么调用
时间: 2024-02-15 15:03:01 浏览: 201
`cursor.fetchall()` 返回的结果是一个元组(tuple)格式的列表(list),其中每个元素都是一条记录,每条记录又是一个由字段值组成的元组(tuple)。你可以使用下标(index)或者字段名(field name)来获取相应的字段值。例如,假设查询结果有两个字段 `id` 和 `name`,并且有两条记录,其中一条记录的 `id` 为 1,`name` 为 "Alice",另一条记录的 `id` 为 2,`name` 为 "Bob",那么你可以按照以下方式获取相应的字段值:
使用下标(index)获取字段值:
```python
import pymysql
# 连接数据库
conn = pymysql.connect(host='localhost', user='root', password='123456', database='test')
# 创建游标对象
cursor = conn.cursor()
# 执行查询
cursor.execute('SELECT id, name FROM users')
# 获取查询结果
results = cursor.fetchall()
# 遍历查询结果,并按照下标(index)获取字段值
for row in results:
id = row[0]
name = row[1]
print(f'id={id}, name={name}')
# 关闭游标和连接
cursor.close()
conn.close()
```
使用字段名(field name)获取字段值:
```python
import pymysql
# 连接数据库
conn = pymysql.connect(host='localhost', user='root', password='123456', database='test')
# 创建游标对象
cursor = conn.cursor()
# 执行查询
cursor.execute('SELECT id, name FROM users')
# 获取查询结果
results = cursor.fetchall()
# 获取字段名列表
fields = [field[0] for field in cursor.description]
# 遍历查询结果,并按照字段名(field name)获取字段值
for row in results:
row_dict = dict(zip(fields, row))
id = row_dict['id']
name = row_dict['name']
print(f'id={id}, name={name}')
# 关闭游标和连接
cursor.close()
conn.close()
```
这里的 `cursor.description` 用于获取查询结果的字段名信息,返回一个元组(tuple)格式的列表(list),其中每个元素都是一个包含字段信息的元组(tuple),每个字段信息又是一个元组(tuple),其中包含字段名、数据类型、是否可为空等信息。通过这个信息,我们可以构造出一个包含字段名和字段值的字典(dict),方便使用字段名(field name)来获取相应的字段值。
阅读全文
相关推荐
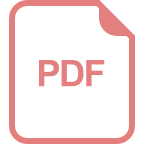
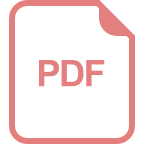
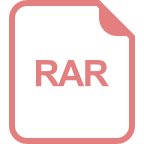















