java抓取网易邮箱邮件基于imap协议 且设置时间范围
时间: 2023-10-03 09:03:59 浏览: 83
要抓取网易邮箱的邮件并设置时间范围,你可以使用JavaMail API来实现。下面是一个基于IMAP协议的示例代码:
```java
import java.util.Properties;
import javax.mail.*;
import javax.mail.internet.*;
public class MailFetcher {
public static void main(String[] args) throws Exception {
String host = "imap.163.com";
String username = "your_email@163.com";
String password = "your_password";
int port = 993;
boolean useSSL = true;
String fromDate = "2022-01-01"; // 要抓取的邮件时间范围起始日期
String toDate = "2022-01-31"; // 要抓取的邮件时间范围结束日期
// 设置连接属性
Properties props = new Properties();
props.put("mail.store.protocol", "imap");
props.put("mail.imap.host", host);
props.put("mail.imap.port", port);
props.put("mail.imap.ssl.enable", useSSL);
// 获取会话
Session session = Session.getInstance(props, new Authenticator() {
@Override
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(username, password);
}
});
// 连接邮箱并打开Folder
Store store = session.getStore("imap");
store.connect();
Folder folder = store.getFolder("INBOX");
folder.open(Folder.READ_ONLY);
// 设置时间范围
SearchTerm searchTerm = new SentDateTerm(ComparisonTerm.GE, new java.util.Date(fromDate));
searchTerm = new AndTerm(searchTerm, new SentDateTerm(ComparisonTerm.LE, new java.util.Date(toDate)));
// 过滤邮件并打印
Message[] messages = folder.search(searchTerm);
for (Message message : messages) {
System.out.println("Subject: " + message.getSubject());
System.out.println("From: " + message.getFrom()[0]);
System.out.println("Sent Date: " + message.getSentDate());
}
// 关闭Folder和Store
folder.close(false);
store.close();
}
}
```
在代码中,我们使用了JavaMail API连接到网易邮箱的IMAP服务器,然后打开收件箱Folder并设置时间范围。我们使用了`SentDateTerm`来设置时间范围,`ComparisonTerm.GE`表示大于等于,`ComparisonTerm.LE`表示小于等于,具体日期可以使用`java.util.Date`来表示。最后,我们过滤出符合时间范围的邮件并打印出来。
需要注意的是,如果你的邮箱开启了两步验证,你需要生成一个授权码来代替密码。另外,如果你的邮箱开启了POP3服务而不是IMAP服务,你需要将代码中的`imap`改为`pop3`。
阅读全文
相关推荐
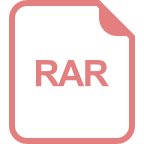
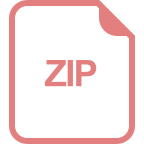
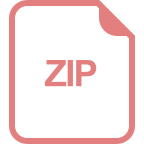
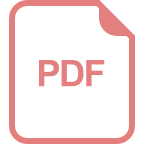
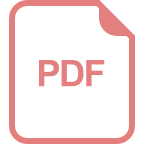
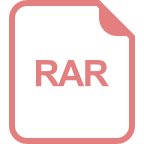
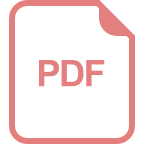
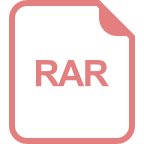
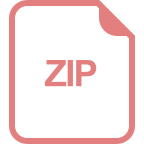
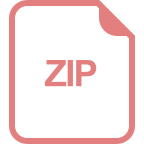
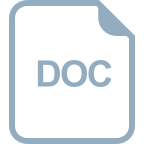
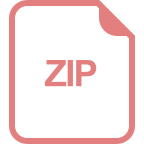
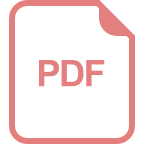
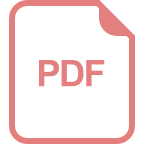
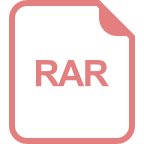
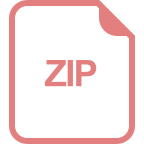
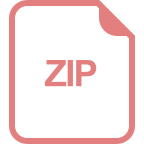
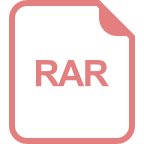